Java点击按钮替换文本文件第二行内容
时间: 2024-06-21 20:03:56 浏览: 9
在Java中,你可以使用Swing或JavaFX等GUI库来创建界面,然后利用文件I/O操作来读取和修改文本文件的内容。下面是一个简单的例子,展示如何创建一个按钮,当点击时会替换文本文件的第二行内容:
```java
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
import java.io.File;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) {
// 创建一个新的窗口
JFrame frame = new JFrame("File Replace");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
// 创建一个面板
JPanel panel = new JPanel();
frame.add(panel);
// 创建一个按钮
JButton button = new JButton("Replace File Line");
button.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String lineToReplace = "New Line Content"; // 要替换成的新内容
try {
// 读取原文件
File file = new File("path_to_your_file.txt"); // 替换为你的文件路径
String content = Files.readAllLines(file.toPath(), StandardCharsets.UTF_8).get(1); // 获取第二行(索引从0开始)
// 替换第二行
content = content.replaceFirst(content, lineToReplace);
// 写回文件
Files.write(file.toPath(), Arrays.asList(content), StandardCharsets.UTF_8);
JOptionPane.showMessageDialog(frame, "Line replaced successfully!");
} catch (IOException ex) {
JOptionPane.showMessageDialog(frame, "Error replacing line: " + ex.getMessage());
}
}
});
panel.add(button);
frame.pack();
frame.setVisible(true);
}
}
```
**相关问题:**
1. Java Swing和JavaFX有什么区别?它们各自适合什么样的场景?
2. 在Java中如何处理文件I/O异常,例如`FileNotFoundException`?
3. 如果文件不存在,这个例子应该如何处理?
相关推荐
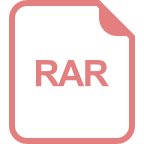
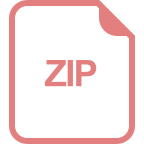
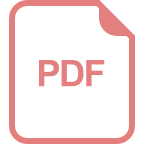











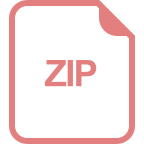