帮我用c++不使用任何库生成一个栈,并用这个栈实现表达式求值的功能
时间: 2024-10-07 16:03:08 浏览: 26
在 C++ 中,你可以使用基本的结构体或模板类来实现一个栈(Stack),这里我们先创建一个简单的栈结构,然后利用栈的数据结构特性来实现一个基于中缀表达式的后序计算,进而求值。
首先,我们需要一个栈结构(这里仅用于存储操作数):
```cpp
#include <stack>
// 定义栈元素(假设只处理整数)
struct StackElement {
int value;
};
// 创建栈类
class CustomStack {
private:
std::stack<StackElement> stack;
public:
// 入栈
void push(int val) { stack.push({val}); }
// 出栈并返回顶部元素
int pop() { return stack.top().value; }
// 判断是否为空
bool empty() const { return stack.empty(); }
};
```
接着,我们将实现一个函数 `evaluate`,它接受一个中缀表达式字符串和栈作为输入,通过后序遍历的方式计算表达式的值:
```cpp
int evaluate(std::string expression, CustomStack& stack) {
// 定义操作符优先级
std::map<char, int> priority = {'+', '-', '*', '/'};
// 临时存储字符
char current_char;
int left_operand = 0, right_operand;
for (size_t i = 0; i < expression.length(); ++i) {
current_char = expression[i];
if (isdigit(current_char)) { // 如果是数字,直接入栈
stack.push(i + 1); // 表示位置索引,因为栈里只放数值,所以加一
} else if (current_char == '(') { // 左括号,忽略
continue;
} else if (current_char == ')') { // 右括号,计算直到找到左括号
while (!stack.empty() && stack.top() != '(') {
right_operand = i;
left_operand = stack.pop();
stack.push(evaluate(expression.substr(left_operand, right_operand - left_operand)));
}
stack.pop(); // 移除左括号
} else { // 操作符,查找其优先级并按需出栈计算
while (!stack.empty() && priority[current_char] <= priority[stack.top().value]) {
right_operand = i;
left_operand = stack.pop();
stack.push(evaluate(expression.substr(left_operand, right_operand - left_operand)));
}
stack.push(current_char); // 将操作符压入栈中
}
}
// 计算剩余的操作数
while (!stack.empty()) {
right_operand = expression.length();
left_operand = stack.pop();
stack.push(evaluate(expression.substr(left_operand, right_operand - left_operand)));
}
return stack.pop(); // 返回最终结果
}
```
现在你可以使用这个栈来计算表达式了,例如:
```cpp
int main() {
CustomStack stack;
std::string expression = "4 * (5 + 7) / 3";
int result = evaluate(expression, stack);
std::cout << "Expression evaluation result: " << result << std::endl;
return 0;
}
```
阅读全文
相关推荐
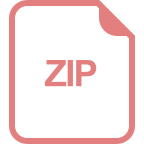
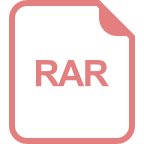
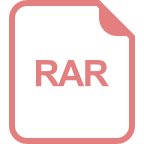
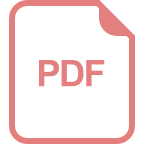
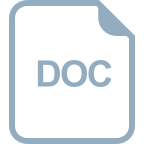
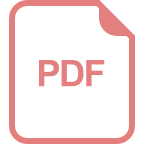
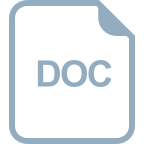
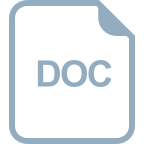
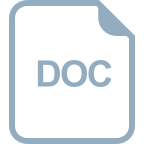
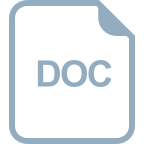
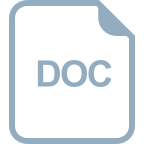
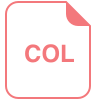
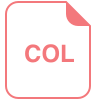
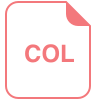
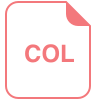
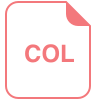
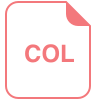
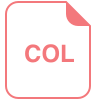
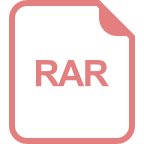