java jsp链接数据库如何实现分页展示
时间: 2024-01-17 11:03:35 浏览: 132
要在Java JSP中实现分页展示,你可以按照以下步骤进行操作:
1. 在JSP页面中,创建一个表格或其他适当的HTML元素来展示数据。
2. 在Java代码中,编写一个方法来连接数据库,并执行查询操作来获取数据。
3. 使用分页算法来确定每页显示的数据范围,并将其应用于查询结果。
4. 在JSP页面中使用循环语句(如`<c:forEach>`标签)来遍历查询结果,并将数据显示在表格中。
5. 创建一个分页导航栏,允许用户切换至不同的页面。
6. 根据用户的选择,更新分页算法的参数,并重新执行查询以获取新的数据。
以下是一个简单的示例代码,演示如何在JSP页面中实现基本的分页展示:
```java
<%-- 在JSP页面中使用JSTL标签库 --%>
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
<%-- 设置每页显示的记录数 --%>
<% int pageSize = 10; %>
<%-- 获取当前页数(从请求参数中获取) --%>
<% int currentPage = Integer.parseInt(request.getParameter("page")); %>
<%-- 计算起始记录的索引 --%>
<% int startIndex = (currentPage - 1) * pageSize; %>
<%-- 连接数据库并执行查询操作 --%>
<%
// 导入必要的数据库连接和查询代码
Connection conn = null;
Statement stmt = null;
ResultSet rs = null;
try {
// 连接数据库
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
stmt = conn.createStatement();
// 执行查询
String query = "SELECT * FROM mytable LIMIT " + startIndex + ", " + pageSize;
rs = stmt.executeQuery(query);
// 在表格中显示查询结果
%>
<table>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
</tr>
<% while (rs.next()) { %>
<tr>
<td><%= rs.getInt("id") %></td>
<td><%= rs.getString("name") %></td>
<td><%= rs.getInt("age") %></td>
</tr>
<% } %>
</table>
<%
} catch (SQLException e) {
e.printStackTrace();
} finally {
// 关闭数据库连接和结果集
if (rs != null) {
try {
rs.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (stmt != null) {
try {
stmt.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
if (conn != null) {
try {
conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
%>
<%-- 创建分页导航栏 --%>
<div>
<%-- 计算总页数 --%>
<% int totalRecords = 100; %>
<% int totalPages = (int) Math.ceil((double) totalRecords / pageSize); %>
<%-- 显示分页导航链接 --%>
<% for (int i = 1; i <= totalPages; i++) { %>
<a href="?page=<%= i %>"><%= i %></a>
<% } %>
</div>
```
请注意,上述代码只是一个简单的示例,仅用于演示如何在JSP页面中实现分页展示。根据你的具体需求,你可能需要进行更多的错误处理、参数验证和优化。同时,为了安全起见,你应该考虑使用预编译语句或对象关系映射(ORM)框架来执行数据库查询操作。
阅读全文
相关推荐
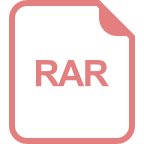
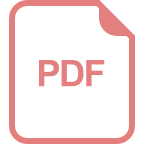
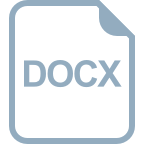
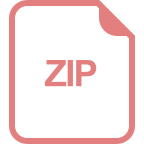
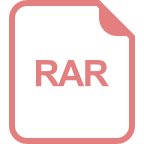
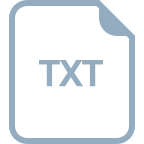
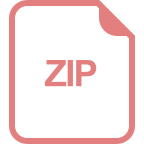
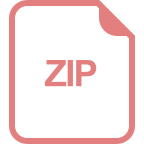
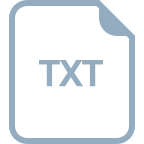
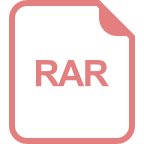
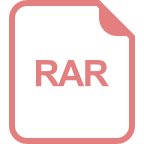
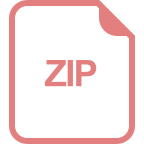
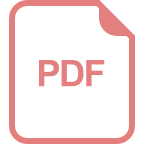
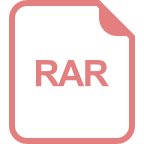
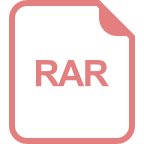
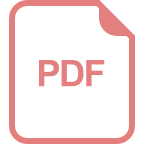