python判断文件中的文件名称是否在本地目录下下
时间: 2024-11-25 14:18:55 浏览: 4
在Python中,你可以使用`os`模块来判断文件名是否存在于当前工作目录下。首先,你需要获取当前目录的路径,然后检查指定的文件名是否在这个目录内。下面是一个简单的例子:
```python
import os
def is_file_in_dir(filename):
current_dir = os.getcwd() # 获取当前工作目录
file_path = os.path.join(current_dir, filename) # 拼接文件路径
if os.path.isfile(file_path): # 使用os.path.isfile()检查是否存在
return True
else:
return False
# 调用函数并打印结果
filename_to_check = "example.txt"
if is_file_in_dir(filename_to_check):
print(f"{filename_to_check}在当前目录下")
else:
print(f"{filename_to_check}不在当前目录下")
相关问题
python如何读取本地文件再在html中打开
在Python中,你可以使用内置的`open()`函数读取本地文件的内容,然后使用模板引擎或者简单的字符串操作将其插入到HTML文件中。以下是一个基本的例子:
```python
# 首先,打开并读取文本文件内容
with open('path_to_your_file.txt', 'r') as file:
content = file.read()
# 然后创建一个新的HTML片段,将内容插入到`<body>`标签中
html_template = f"""
<!DOCTYPE html>
<html>
<head>
<title>File Content</title>
</head>
<body>
<p>{content}</p>
</body>
</html>
"""
# 将生成的HTML保存到新的文件中
with open('output.html', 'w') as output_file:
output_file.write(html_template)
```
在这个例子中,你需要替换`'path_to_your_file.txt'`为你要读取的实际文件路径。生成的`output.html`文件将在当前工作目录下创建,并包含从txt文件读取的数据。
python读取文件目录中的h5 文件
你可以使用`os`模块和`h5py`库来读取文件目录中的h5文件。以下是一个示例代码:
```python
import os
import h5py
# 指定文件夹路径
folder_path = '/path/to/folder'
# 遍历文件夹中的文件
for filename in os.listdir(folder_path):
# 判断文件是否为h5文件
if filename.endswith('.h5'):
# 打开h5文件
with h5py.File(os.path.join(folder_path, filename), 'r') as f:
# 读取数据
data = f['dataset_name'][:] # dataset_name为h5文件中数据集的名称
# 处理数据
# ...
```
代码中,首先使用`os.listdir`函数遍历指定文件夹中的所有文件,然后判断文件是否为h5文件。如果是h5文件,就使用`h5py.File`打开文件,并读取指定数据集的数据。最后可以对数据进行处理。
阅读全文
相关推荐
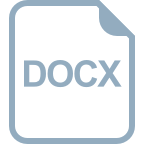
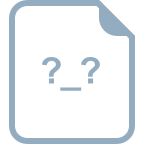
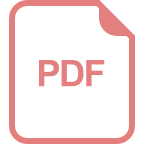













