C#路径前加\\?\起到什么作用
时间: 2023-12-22 20:29:51 浏览: 25
C#路径前加`\\?\`可以改变路径解析的方式,它的作用有以下几点:
1. 绕过路径长度限制:在Windows中,路径的最大长度是260个字符。但是,通过在路径前加上`\\?\`,可以绕过这个限制,允许处理长路径。
2. 支持特殊字符:在路径中使用特殊字符(如空格、句点等)时,可能会导致路径解析错误。通过在路径前加上`\\?\`,可以确保这些特殊字符被正确解析。
3. 支持UNC路径:UNC路径是指以双斜杠`\\`开头的网络共享路径。在某些情况下,使用UNC路径可能会导致路径解析错误。通过在UNC路径前加上`\\?\UNC\`,可以确保UNC路径被正确解析。
下面是一个示例代码,演示了在C#中使用`\\?\`前缀的路径:
```csharp
using System;
using System.IO;
public class PathExample
{
public static void Main()
{
string path = @"\\?\C:\very\long\path\to\a\file.txt";
string fullPath = Path.GetFullPath(path);
Console.WriteLine(fullPath);
}
}
```
运行结果:
```
C:\very\long\path\to\a\file.txt
```
相关问题
c# ??和??=的例子
以下是C#中 ?? 和 ??= 运算符的例子:
1. ?? 运算符的例子:
```csharp
string str1 = null;
string str2 = str1 ?? "default value";
Console.WriteLine(str2); // 输出 "default value"
string str3 = "C#";
string str4 = str3 ?? "default value";
Console.WriteLine(str4); // 输出 "C#"
```
上述代码中,第一个例子中 str1 为 null,所以使用 ?? 运算符返回了默认值 "default value";第二个例子中 str3 不为 null,所以返回了原始值 "C#"。
2. ??= 运算符的例子:
```csharp
int? num1 = null;
num1 ??= 10;
Console.WriteLine(num1); // 输出 10
int? num2 = 20;
num2 ??= 10;
Console.WriteLine(num2); // 输出 20
```
上述代码中,第一个例子中 num1 为 null,所以使用 ??= 运算符将 num1 赋值为 10;第二个例子中 num2 不为 null,所以不会执行赋值操作,num2 的值仍为 20。
C,C++,C#有什么区别?
C、C++和C#是三种不同的编程语言,它们有以下区别:
1. C语言是一种过程式编程语言,它注重对底层硬件的直接操作和高性能。C语言具有简洁、高效和灵活的特点,广泛应用于系统编程和嵌入式开发领域。
2. C++是在C语言基础上发展起来的一种面向对象编程语言。它在C语言的基础上添加了类、对象、继承、多态等面向对象的特性,同时仍然保留了C语言的底层编程能力。C++具有强大的功能和广泛的应用领域,包括游戏开发、图形界面设计等。
3. C#(读作C Sharp)是微软公司开发的一种面向对象的编程语言。它结合了C++和Java的特点,并在.NET框架下运行。C#具有简单易学、安全性高、跨平台等特点,广泛应用于Windows平台的软件开发。
总结来说,C语言注重底层和性能,C++在C语言基础上添加了面向对象的特性,而C#则是微软公司开发的一种面向对象编程语言。选择使用哪种语言主要取决于具体的应用场景和个人偏好。
相关推荐
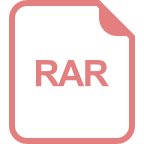
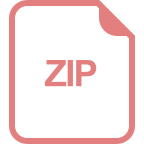
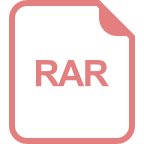












