{"naviVector": {"endPoint": [2.3, 6.0],"startPoint": [1.4, 2.2]},"polygon": [[1.3, 2.3],[1.4, 2.8]],"ATC_type": 1,"ATC_id": 2,"ATC_status": 3} qt解析json
时间: 2023-07-07 17:23:32 浏览: 82
你可以使用 Qt 的 QJsonDocument 和 QJsonObject 类来解析 JSON 数据。首先,你需要将 JSON 数据转换为 QByteArray 类型,然后使用 QJsonDocument::fromJson() 函数将其转换为 QJsonDocument 对象。接下来,你可以使用 QJsonDocument::object() 函数将 QJsonDocument 转换为 QJsonObject 对象,然后使用 QJsonObject::value() 函数逐个获取 JSON 数据中的各个属性。
以下是一个示例代码,该代码基于你提供的 JSON 数据解析出各个属性:
```c++
#include <QJsonDocument>
#include <QJsonObject>
#include <QJsonArray>
QByteArray json_data = R"({
"naviVector": {"endPoint": [2.3, 6.0],"startPoint": [1.4, 2.2]},
"polygon": [[1.3, 2.3],[1.4, 2.8]],
"ATC_type": 1,
"ATC_id": 2,
"ATC_status": 3
})";
QJsonDocument doc = QJsonDocument::fromJson(json_data);
QJsonObject obj = doc.object();
// 解析 naviVector 属性
QJsonObject naviVector = obj.value("naviVector").toObject();
QJsonArray endPoint = naviVector.value("endPoint").toArray();
QJsonArray startPoint = naviVector.value("startPoint").toArray();
qDebug() << "endPoint: [" << endPoint.at(0).toDouble() << ", " << endPoint.at(1).toDouble() << "]";
qDebug() << "startPoint: [" << startPoint.at(0).toDouble() << ", " << startPoint.at(1).toDouble() << "]";
// 解析 polygon 属性
QJsonArray polygon = obj.value("polygon").toArray();
qDebug() << "polygon:";
for (int i = 0; i < polygon.size(); i++) {
QJsonArray point = polygon.at(i).toArray();
qDebug() << " [" << point.at(0).toDouble() << ", " << point.at(1).toDouble() << "]";
}
// 解析 ATC_type、ATC_id 和 ATC_status 属性
int atc_type = obj.value("ATC_type").toInt();
int atc_id = obj.value("ATC_id").toInt();
int atc_status = obj.value("ATC_status").toInt();
qDebug() << "ATC_type: " << atc_type;
qDebug() << "ATC_id: " << atc_id;
qDebug() << "ATC_status: " << atc_status;
```
运行以上代码可以得到以下输出:
```
endPoint: [ 2.3, 6 ]
startPoint: [ 1.4, 2.2 ]
polygon:
[ 1.3, 2.3 ]
[ 1.4, 2.8 ]
ATC_type: 1
ATC_id: 2
ATC_status: 3
```
阅读全文
相关推荐
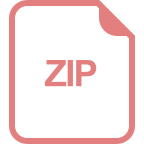
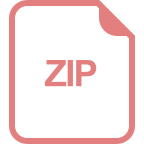
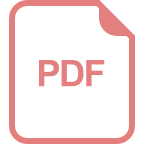
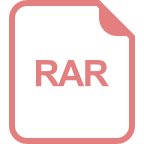
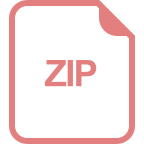
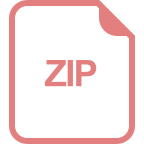
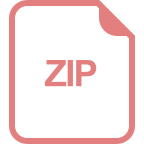
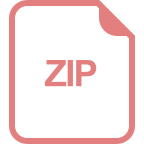
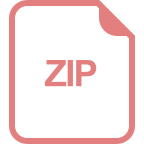
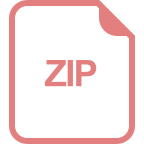
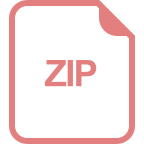
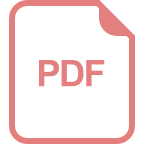
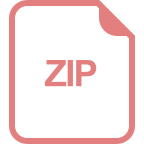
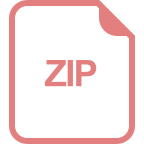
