c#接口使用
时间: 2023-10-15 12:08:09 浏览: 42
C#中的接口是一种契约,它定义了一个类应该实现哪些方法和属性。 接口可以被用于多态性,也可以用于实现类之间的协作。下面是一个简单的接口示例:
```csharp
public interface IShape
{
double GetArea();
double GetPerimeter();
}
public class Circle : IShape
{
private double radius;
public Circle(double radius)
{
this.radius = radius;
}
public double GetArea()
{
return Math.PI * radius * radius;
}
public double GetPerimeter()
{
return 2 * Math.PI * radius;
}
}
public class Rectangle : IShape
{
private double length;
private double width;
public Rectangle(double length, double width)
{
this.length = length;
this.width = width;
}
public double GetArea()
{
return length * width;
}
public double GetPerimeter()
{
return 2 * (length + width);
}
}
```
在这个示例中,IShape接口定义了两个方法GetArea和GetPerimeter。Circle和Rectangle类都实现了这个接口,并提供了自己的实现。这样,我们就可以使用多态性来处理这些对象:
```csharp
IShape[] shapes = new IShape[2];
shapes[0] = new Circle(5);
shapes[1] = new Rectangle(4, 6);
foreach (IShape shape in shapes)
{
Console.WriteLine("Area: {0}", shape.GetArea());
Console.WriteLine("Perimeter: {0}", shape.GetPerimeter());
}
```
输出:
```
Area: 78.53981633974483
Perimeter: 31.41592653589793
Area: 24
Perimeter: 20
```
在这个示例中,我们使用IShape接口的引用来处理Circle和Rectangle对象。这使我们能够使用相同的代码来处理不同类型的对象。
相关推荐
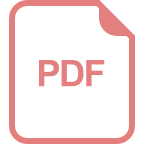
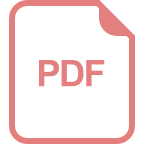
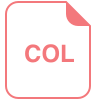
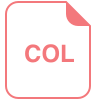
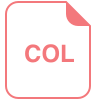
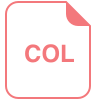
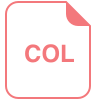









