C语言输入一系列英文单词,单词之间用空格隔开(一到多个空格),用“###”表示输入结束,统计输入过哪些单词以及各单词出现的次数,统计时区分大小写字母。本题假定:不重复的单词数不超过100个;每个单词长度限制在20以内。 输入: 占一行 输出: 占一行,其格式为:单词1-个数 单词2-个数 …(单词与个数中间用减号连接,各输出组之间用一个空格分隔,单词依照出现的先后次序统计) 样例: 输入:aaa bbb aaa ### 输出:aaa-2 bbb-1
时间: 2023-06-26 16:06:28 浏览: 850
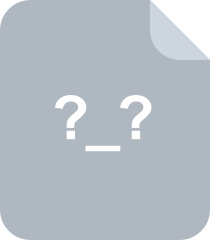
C语言程序设计-将从键盘上输入的每个单词的第一个字母转换为大写字母,输入时各单词必须用空格隔开,用’.’结束输入; .c
以下是一个可能的C语言代码实现,使用了哈希表统计单词出现次数:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_WORD_LEN 20
#define MAX_WORDS 100
typedef struct {
char word[MAX_WORD_LEN + 1];
int count;
} WordCount;
typedef struct {
WordCount entries[MAX_WORDS];
int size;
} WordMap;
int hash(const char *s) {
int h = 0;
while (*s) {
h = (h * 31 + *s) % MAX_WORDS;
s++;
}
return h;
}
int find_word(WordMap *map, const char *word) {
int h = hash(word);
while (map->entries[h].count > 0) {
if (strcmp(map->entries[h].word, word) == 0) {
return h;
}
h = (h + 1) % MAX_WORDS;
}
return h;
}
void add_word(WordMap *map, const char *word) {
int index = find_word(map, word);
if (map->entries[index].count == 0) {
strcpy(map->entries[index].word, word);
map->entries[index].count = 1;
map->size++;
} else {
map->entries[index].count++;
}
}
int main() {
char line[1024];
WordMap map = {0};
fgets(line, sizeof(line), stdin); // 读入一行输入
while (strcmp(line, "###\n") != 0) {
char *p = line;
while (*p) {
char word[MAX_WORD_LEN + 1] = "";
int i = 0;
while (*p && *p != ' ' && i < MAX_WORD_LEN) {
word[i++] = *p;
p++;
}
while (*p == ' ') {
p++;
}
add_word(&map, word);
}
fgets(line, sizeof(line), stdin);
}
for (int i = 0; i < map.size; i++) {
printf("%s-%d", map.entries[i].word, map.entries[i].count);
if (i < map.size - 1) {
printf(" ");
}
}
printf("\n");
return 0;
}
```
该程序使用 `fgets` 函数读入一行输入,然后在循环中逐个提取单词,并使用哈希表统计单词出现次数。最后输出哈希表中所有非空的单词和对应的出现次数。注意,题目要求输入以字符串 "###\n" 结尾,因此需要检查输入行是否为 "###\n",以判断输入是否结束。
阅读全文
相关推荐
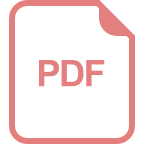
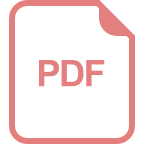
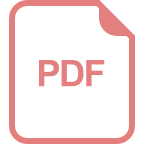
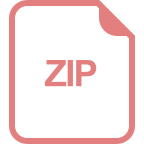
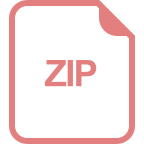
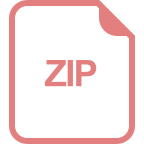
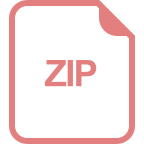
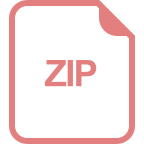