使用QThread制作实现:2. 读取的文件显示在主窗口的两个QLabel当中 3. 开始按钮 实现启动线程,开始更新图片 4. 暂停按钮 实现图片暂停刷新
时间: 2024-02-03 16:13:31 浏览: 84
下面是使用QThread实现读取文件并在主窗口显示的代码:
```python
from PyQt5.QtCore import QThread, pyqtSignal
from PyQt5.QtGui import QPixmap
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton
import time
class ReadFileThread(QThread):
update_image = pyqtSignal(QPixmap)
def __init__(self, file_path):
super().__init__()
self.file_path = file_path
self.is_running = True
def run(self):
while self.is_running:
pixmap = QPixmap(self.file_path)
self.update_image.emit(pixmap)
time.sleep(1)
def stop(self):
self.is_running = False
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.label1 = QLabel(self)
self.label2 = QLabel(self)
self.label1.setGeometry(10, 10, 300, 300)
self.label2.setGeometry(320, 10, 300, 300)
self.start_button = QPushButton('开始', self)
self.start_button.setGeometry(10, 320, 100, 30)
self.start_button.clicked.connect(self.start_thread)
self.pause_button = QPushButton('暂停', self)
self.pause_button.setGeometry(120, 320, 100, 30)
self.pause_button.clicked.connect(self.pause_thread)
self.read_file_thread = None
def start_thread(self):
if self.read_file_thread is None:
self.read_file_thread = ReadFileThread('file.jpg')
self.read_file_thread.update_image.connect(self.update_image)
self.read_file_thread.start()
def pause_thread(self):
if self.read_file_thread is not None:
self.read_file_thread.stop()
self.read_file_thread = None
def update_image(self, pixmap):
self.label1.setPixmap(pixmap)
self.label2.setPixmap(pixmap)
if __name__ == '__main__':
app = QApplication([])
window = MainWindow()
window.setGeometry(100, 100, 640, 360)
window.show()
app.exec_()
```
其中,`ReadFileThread`是一个继承自`QThread`的类,用于读取文件并更新图片。在`run`方法中,不断读取文件并通过`update_image`信号将`QPixmap`对象传递给主窗口。`stop`方法用于停止线程。
`MainWindow`类中有两个`QLabel`用于显示图片,以及两个按钮用于开始和暂停线程。`start_thread`方法用于创建并启动`ReadFileThread`对象,`pause_thread`方法用于停止线程。`update_image`方法用于更新图片。
在主程序中,创建`QApplication`和`MainWindow`对象,设置窗口大小并显示窗口。
阅读全文
相关推荐
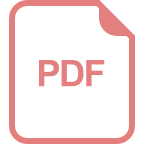
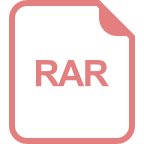
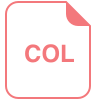
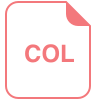
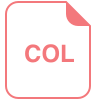
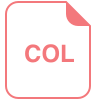
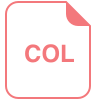
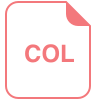
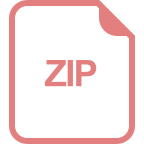
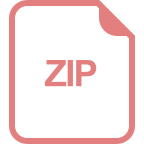
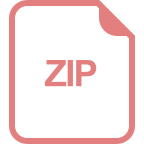
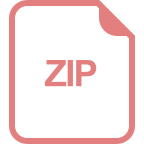