C++ 与QT 用于将两个字节(byte数组)转换为一个 16 位的整数(Int16)函数
时间: 2024-10-25 13:04:55 浏览: 41
在C++中,特别是使用Qt这样的框架,你可以使用内置的数据类型和一些基本的数学运算来将两个字节的数组转换成一个16位整数(Int16)。这里有一个简单的示例:
```cpp
#include <QByteArray>
#include <qint16>
// 假设我们有两个字节的数组bytes,例如从网络接收或硬盘读取
QByteArray bytes = QByteArray({0x01, 0x02}); // 这代表十六进制的0x0102
// 使用QByteArray的toShort()函数将字节转换为Int16
qint16 int16Value = bytes.toShort();
```
`toShort()`函数会将字节数组的第一个元素作为最低位,第二个元素作为次低位,合并成一个16位整数。如果你需要反向顺序(即高位在前),可以在提取字节之前先颠倒字节数组:
```cpp
// 颠倒字节顺序
QByteArray reversedBytes = bytes.mid(1, 1) + bytes.left(1);
int16Value = reversedBytes.toShort();
```
但是请注意,对于大多数现代计算机体系结构,字节通常是从低到高存储的,所以原始的`toShort()`应该足以得到正确的结果。
阅读全文
相关推荐
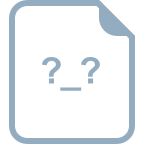
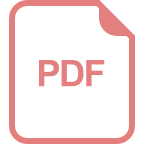
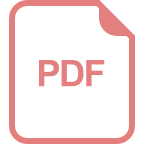
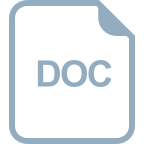
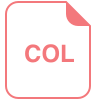
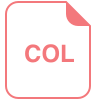
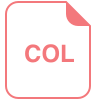
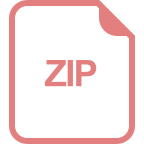
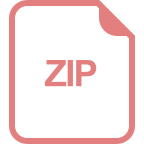
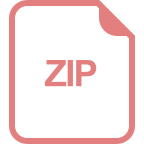
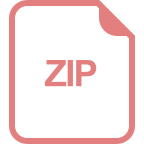
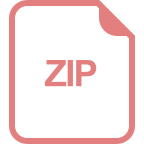
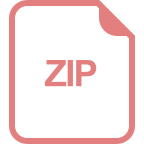
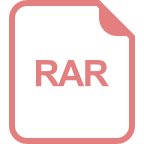
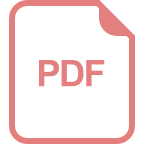
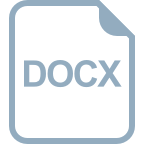
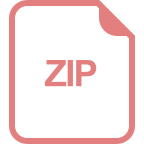