给定一个字符串,编写一个程序来进行大小写转换,并将字符串中的每个单词的首字母大写python
时间: 2024-09-24 09:25:11 浏览: 70
在Python中,你可以使用内置的`title()`函数来完成字符串的标题化,也就是将每个单词的首字母大写。如果你想自己编写一个简单的函数,可以遍历每个字符并检查是否为单词的开头,如果是,则将其转换为大写。以下是这两种方法的示例:
1. 使用内置 `title()` 函数:
```python
def title_case(s):
return s.title()
input_string = "hello world"
converted_string = title_case(input_string)
print(converted_string) # 输出: Hello World
```
2. 手动实现转换:
```python
def manual_title_case(s):
words = s.split(' ')
capitalized_words = [word[0].upper() + word[1:] for word in words]
return ' '.join(capitalized_words)
input_string = "hello world"
converted_string_manual = manual_title_case(input_string)
print(converted_string_manual) # 输出: Hello World
```
相关问题
C语言给定一个字符串,将字符串中每个单词大小写逐步翻转
在C语言中,你可以通过遍历字符串、检查字符的ASCII码值以及利用条件语句来实现单词大小写的逐步翻转。以下是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h> // 包含处理字母大小写的函数
void reverse_case(char *str) {
int i = 0;
while (str[i]) { // 遍历字符串直到遇到空字符
if (isalpha(str[i])) { // 如果字符是字母
if (islower(str[i])) { // 如果是小写字母
str[i] = toupper(str[i]); // 转换为大写
} else { // 否则是大写字母
str[i] = tolower(str[i]); // 转换为小写
}
}
i++;
}
}
int main() {
char input[] = "Hello World! This is a Test.";
printf("Original String: %s\n", input);
reverse_case(input);
printf("Flipped String: %s\n", input); // 输出结果会逐步反转单词的大小写
return 0;
}
```
在这个程序中,`reverse_case` 函数接收一个字符串指针作为输入,然后逐个检查每个字符,如果是字母则转换大小写。`main` 函数中提供了一个测试字符串,并展示了如何使用这个函数。
用python写一个程序:给定某一字符串s,对其中的每一字符C大小写转换:如果c是大写字母,则将它转换成小写字母;如果c是小写字母,则将它转换成大写字母:如果c不是字母,则不转换。
以下是用 Python 编写的程序,可以实现对字符串中每个字符的大小写转换:
```python
def convert_case(s):
result = ""
for c in s:
if c.isalpha():
if c.isupper():
result += c.lower()
else:
result += c.upper()
else:
result += c
return result
```
你可以将需要转换的字符串作为参数传递给 `convert_case` 函数,它将返回转换后的字符串。例如,如果你想将字符串 `"Hello, World!"` 中的每个字符大小写互换,可以这样调用函数:
```python
s = "Hello, World!"
result = convert_case(s)
print(result)
```
输出结果为:
```
hELLO, wORLD!
```
阅读全文
相关推荐
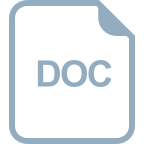
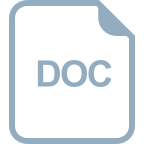
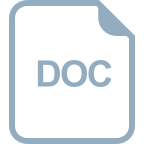













