vue3中页面有两个div,实现按下鼠标后拖动左侧div边框调整左右两侧div的宽度
时间: 2023-11-21 19:06:19 浏览: 167
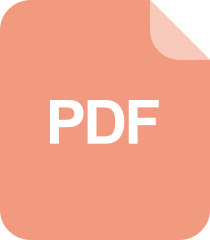
vue实现div可拖动位置也可改变盒子大小的原理

可以使用vue3的指令来实现这个功能。具体实现步骤如下:
1. 在左侧div中添加mousedown事件监听器,当鼠标按下时触发事件。
2. 在整个页面中添加mousemove和mouseup事件监听器,当鼠标移动时触发mousemove事件,当鼠标松开时触发mouseup事件。
3. 在mousedown事件处理程序中,记录左侧div的初始宽度、鼠标按下时的位置和整个页面的宽度。
4. 在mousemove事件处理程序中,根据鼠标移动的距离计算出左侧div的新宽度,并设置左侧div的宽度和右侧div的宽度。
5. 在mouseup事件处理程序中,移除mousemove和mouseup事件监听器。
具体实现代码如下:
```
<template>
<div class="container">
<div class="left" :style="{ width: leftWidth + 'px' }" @mousedown="onMouseDown"></div>
<div class="right"></div>
</div>
</template>
<script>
export default {
data() {
return {
leftWidth: 200, // 左侧div的初始宽度
mouseX: 0, // 鼠标按下时的位置
pageWidth: 0 // 整个页面的宽度
}
},
mounted() {
this.pageWidth = document.body.clientWidth
},
methods: {
onMouseDown(event) {
this.mouseX = event.clientX
document.addEventListener('mousemove', this.onMouseMove)
document.addEventListener('mouseup', this.onMouseUp)
},
onMouseMove(event) {
const distance = event.clientX - this.mouseX
const newLeftWidth = this.leftWidth + distance
const newRightWidth = this.pageWidth - newLeftWidth
this.leftWidth = newLeftWidth
this.$nextTick(() => {
this.$emit('resize', newLeftWidth, newRightWidth)
})
},
onMouseUp() {
document.removeEventListener('mousemove', this.onMouseMove)
document.removeEventListener('mouseup', this.onMouseUp)
}
}
}
</script>
<style>
.container {
display: flex;
height: 100vh;
}
.left {
height: 100%;
background-color: #eee;
cursor: col-resize;
}
.right {
flex: 1;
height: 100%;
background-color: #fff;
}
</style>
```
在上面的代码中,左侧div的宽度使用响应式数据leftWidth来绑定。鼠标按下事件使用onMouseDown方法来处理,该方法会记录鼠标按下时的位置和整个页面的宽度。鼠标移动事件使用onMouseMove方法来处理,该方法会根据鼠标移动的距离计算出左侧div的新宽度,并设置左侧div的宽度和右侧div的宽度。鼠标松开事件使用onMouseUp方法来处理,该方法会移除mousemove和mouseup事件监听器。
阅读全文
相关推荐
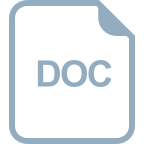
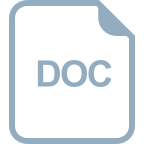

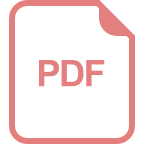


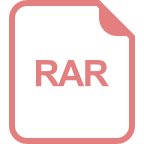
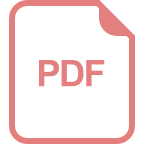
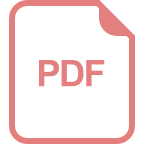





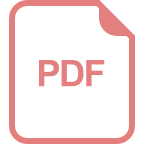
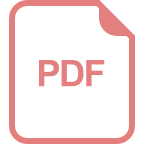
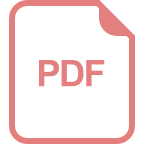
