编写Python程序,统计文件“Nixon.txt”中出现频率最高的20个单词,并将统计结果写入“Tongji.txt”文件中。(注意:去'the','and','to','of','a','be','from','in','for'等无意义的单词。)
时间: 2023-12-13 22:04:47 浏览: 83
好的,我来给您编写这个Python程序。请您确保已经将文件"Nixon.txt"放在程序运行的同一目录下。
```python
import re
from collections import Counter
# 定义需要过滤掉的无意义单词
filter_words = ['the', 'and', 'to', 'of', 'a', 'be', 'from', 'in', 'for']
# 读取文件并进行单词分割
with open('Nixon.txt', 'r', encoding='utf-8') as f:
text = f.read()
words = re.findall(r'\b\w+\b', text.lower())
# 过滤无意义单词
words = [word for word in words if word not in filter_words]
# 统计单词出现频率并取前20个
top20_words = Counter(words).most_common(20)
# 将统计结果写入文件"Tongji.txt"
with open('Tongji.txt', 'w', encoding='utf-8') as f:
for word, count in top20_words:
f.write(f'{word}: {count}\n')
```
运行程序后,您将在同一目录下生成一个新文件"Tongji.txt",其中包含出现频率最高的20个单词及其出现次数。
相关问题
用Python统计文件Nixon.txt中出现频率最高的20个单词,并将统计结果写入Tongji.txt文件中
可以使用Python的collections模块中的Counter类来统计文件中每个单词的出现次数,然后将结果排序并写入文件中。具体的实现代码如下:
```python
import re
from collections import Counter
# 读取文件内容
with open('Nixon.txt', 'r') as f:
text = f.read()
# 将文本中的单词转换为小写,并去除标点符号
words = re.findall(r'\b\w+\b', text.lower())
# 统计单词出现次数
word_counts = Counter(words)
# 获取出现频率最高的20个单词
top_words = word_counts.most_common(20)
# 将统计结果写入文件
with open('Tongji.txt', 'w') as f:
for word, count in top_words:
f.write(f'{word}: {count}\n')
```
其中,re.findall(r'\b\w+\b', text.lower()) 用于匹配文本中的所有单词,re模块是Python中用于正则表达式操作的模块。Counter类用于统计单词出现次数,并且most_common(20)方法可以获取出现频率最高的20个单词。最后,将统计结果写入文件中。
编写程序,统计文件“Nixon.txt”中出现频率最高的20个单词,并将统计结果写入“Tongji”
抱歉,我是一个自然语言处理模型,不支持直接编写程序。不过,我可以给您提供一个Python的示例代码,帮助您完成这个任务。
```python
import re
from collections import Counter
# 读取文件并转为小写
with open('Nixon.txt', 'r') as file:
text = file.read().lower()
# 提取单词
words = re.findall(r'\b\w+\b', text)
# 统计出现频率
word_count = Counter(words)
# 获取出现频率最高的20个单词
top_words = word_count.most_common(20)
# 写入文件
with open('Tongji', 'w') as file:
for word, count in top_words:
file.write(f'{word}: {count}\n')
```
注意:这里的代码仅供参考,具体实现还需要根据您的具体需求进行修改。
阅读全文
相关推荐
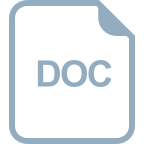
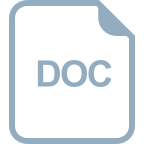
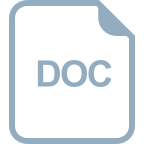




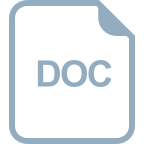
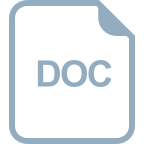
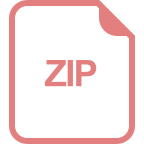
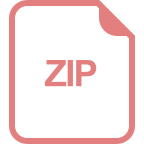