在上面的答案中,如果我是在vue3中使用require,会提示require is not defined,如何解决
时间: 2024-03-04 16:51:48 浏览: 22
在Vue 3中,由于默认情况下使用了ES module,因此不能直接使用CommonJS的require函数,需要使用import来加载模块。
一个解决方法是将图片转为base64编码,然后作为数据绑定到img的src属性上,如下所示:
```
<template>
<div>
<img :src="imageSrc" alt="">
</div>
</template>
<script>
import example from '@/assets/images/example.jpg'
export default {
data() {
return {
imageSrc: ''
}
},
mounted() {
this.imageSrc = `data:image/jpeg;base64,${example}`
}
}
</script>
```
在上面的代码中,首先通过import语句加载图片,然后在mounted钩子函数中将图片转为base64编码,并将其赋值给imageSrc属性,最后将imageSrc绑定到img的src属性上。
当然,如果你不想手动转换图片编码,也可以使用第三方的loader来自动完成这个过程,例如url-loader、base64-loader等。
相关问题
vue3 ts中require is not defined
在Vue 3中使用TypeScript时,如果你在代码中使用了`require`语句,会出现"require is not defined"的错误。这是因为在Vue 3中,不再支持CommonJS的`require`语法,而是使用了ES模块化的`import`语法。
要解决这个问题,你可以将`require`语句替换为`import`语句。例如,如果你想引入一个模块,可以使用以下方式:
```typescript
import moduleName from 'modulePath';
```
如果你需要引入一个具体的文件,可以使用以下方式:
```typescript
import { functionName } from './filePath';
```
另外,如果你需要在Vue组件中使用某个模块或文件,可以在`<script>`标签中使用`import`语句进行引入。
require is not defined vue3
在Vue 3中,使用require方法会报错"require is not defined"。这是因为在Vue 3中,已经不再支持使用CommonJS的require语法进行模块导入。相反,Vue 3使用了ES模块的import语法进行模块导入。
解决这个问题的方法是使用新的方法,例如使用import.meta.globEager来导入资源文件。具体来说,可以使用以下代码来导入指定目录下的所有png文件:
import.meta.globEager("../../assets/authentication/*.png") [2]
需要注意的是,上述代码是使用Vite构建工具的方式进行的,因为Vite是Vue 3官方推荐的构建工具。具体的使用方法可以查看Vite官网上的文档。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [vue3中require报错 require is not defined](https://blog.csdn.net/lys20000913/article/details/122959885)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *3* [ReferenceError: require is not defined 【vue3 +vite +setup语法使用报错】](https://blog.csdn.net/qq_43813351/article/details/129465422)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
相关推荐
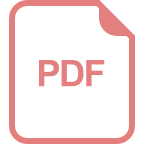
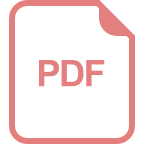












