java调用打印机打印入库单
时间: 2023-08-12 22:09:00 浏览: 59
Java调用打印机打印入库单的具体实现方式需要根据你所使用的打印机和打印模板来确定。以下是一般的实现步骤:
1. 创建入库单对象,包含所需的入库信息。
2. 根据入库单对象填充打印模板,生成打印数据。
3. 使用JasperReports或其他报表工具将打印数据生成为可打印的格式。
4. 使用Java打印库将打印数据发送到打印机并进行打印。
以下是一个简单的示例代码:
```java
import java.io.File;
import java.util.HashMap;
import java.util.Map;
import javax.print.PrintService;
import javax.print.PrintServiceLookup;
import net.sf.jasperreports.engine.JasperFillManager;
import net.sf.jasperreports.engine.JasperPrint;
import net.sf.jasperreports.engine.JasperReport;
import net.sf.jasperreports.engine.util.JRLoader;
public class PrintInvoice {
public static void main(String[] args) throws Exception {
// Step 1: create invoice object
Invoice invoice = new Invoice("INV-1234", "John Doe", "2021-01-01", 1000.0);
// Step 2: fill invoice data into print template
Map<String, Object> params = new HashMap<>();
params.put("invoice", invoice);
File templateFile = new File("template/invoice_template.jasper");
JasperReport report = (JasperReport) JRLoader.loadObject(templateFile);
JasperPrint print = JasperFillManager.fillReport(report, params);
// Step 3: print invoice
PrintService[] services = PrintServiceLookup.lookupPrintServices(null, null);
if (services.length > 0) {
PrintService printService = services[0];
print.setPrinterName(printService.getName());
JasperPrintManager.printReport(print, false);
}
}
}
class Invoice {
private String number;
private String customerName;
private String date;
private double amount;
public Invoice(String number, String customerName, String date, double amount) {
this.number = number;
this.customerName = customerName;
this.date = date;
this.amount = amount;
}
public String getNumber() {
return number;
}
public String getCustomerName() {
return customerName;
}
public String getDate() {
return date;
}
public double getAmount() {
return amount;
}
}
```
这个示例代码包含了以下步骤:
1. 创建入库单对象。
2. 根据入库单对象填充打印模板。
3. 使用JasperReports将打印数据生成为可打印的格式。
4. 使用Java打印库获取可用打印机列表并选择第一个打印机,将打印数据发送到打印机并进行打印。
注意:在运行这个示例代码的时候,需要将打印模板的路径和打印机的名称替换为实际的路径和名称。
相关推荐











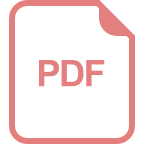
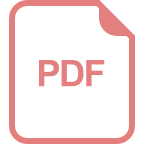
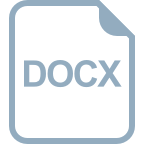