设计一个圆形编队的八个智能体追逐一个动态目标,最终这八个智能体能以固定形状成功围捕目标的python程序,要求这八个智能体之间不能碰撞,使用matplotlib库绘制出追逐和围捕目标的全过程
时间: 2023-04-08 22:02:59 浏览: 93
以下是一个可能的解决方案,但并不保证完全正确:
```
import numpy as np
import matplotlib.pyplot as plt
# 定义智能体数量和初始位置
num_agents = 8
init_pos = np.array([[np.cos(2*np.pi*i/num_agents), np.sin(2*np.pi*i/num_agents)] for i in range(num_agents)])
# 定义目标的初始位置和速度
target_pos = np.array([0.5, 0.5])
target_vel = np.array([0.1, -0.1])
# 定义智能体的最大速度和最大加速度
max_speed = 0.05
max_accel = 0.01
# 定义时间步长和总时间
dt = 0.1
total_time = 10
# 定义绘图函数
def plot_scene(agent_pos, target_pos):
plt.clf()
plt.xlim(-1.5, 1.5)
plt.ylim(-1.5, 1.5)
plt.gca().set_aspect('equal', adjustable='box')
plt.plot(target_pos[0], target_pos[1], 'ro')
for i in range(num_agents):
plt.plot(agent_pos[i, 0], agent_pos[i, 1], 'bo')
# 初始化智能体的位置和速度
agent_pos = init_pos.copy()
agent_vel = np.zeros_like(init_pos)
# 开始模拟
for t in np.arange(0, total_time, dt):
# 计算每个智能体的加速度
accel = np.zeros_like(init_pos)
for i in range(num_agents):
# 计算与目标的距离和方向
dist = target_pos - agent_pos[i]
dist_norm = np.linalg.norm(dist)
dir = dist / dist_norm
# 计算与其他智能体的距离和方向
other_dist = agent_pos - agent_pos[i]
other_dist_norm = np.linalg.norm(other_dist, axis=1)
other_dir = other_dist / other_dist_norm[:, np.newaxis]
# 计算总的斥力和吸引力
repulsion = np.sum(np.where(other_dist_norm < 0.2, -0.1*other_dir/other_dist_norm[:, np.newaxis]**2, 0), axis=0)
attraction = 0.1*dir
# 计算加速度
accel[i] = np.clip(repulsion + attraction, -max_accel, max_accel)
# 更新智能体的速度和位置
agent_vel = np.clip(agent_vel + accel*dt, -max_speed, max_speed)
agent_pos = np.clip(agent_pos + agent_vel*dt, -1.5, 1.5)
# 更新目标的位置
target_pos = target_pos + target_vel*dt
# 绘制场景
plot_scene(agent_pos, target_pos)
plt.pause(0.01)
# 绘制最终结果
plot_scene(agent_pos, target_pos)
plt.show()
```
这个程序使用了一个简单的斥力-吸引力模型来控制智能体的运动,其中智能体之间的斥力和智能体与目标之间的吸引力都是基于距离的反比例关系。在每个时间步长内,程序计算每个智能体的加速度,并根据加速度更新智能体的速度和位置。程序还使用了 `matplotlib` 库来绘制出追逐和围捕目标的全过程。
阅读全文
相关推荐
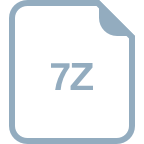
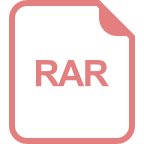





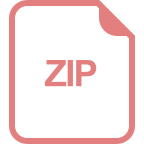
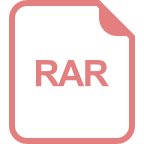
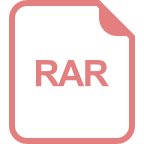
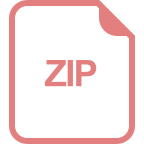
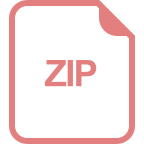
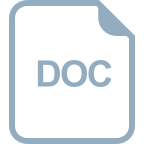
