生产者消费者pv操作c语言
时间: 2023-09-07 22:17:25 浏览: 239
生产者消费者问题是经典的同步问题,可以使用pv操作(信号量)来解决。下面是一个使用pv操作实现生产者消费者问题的C语言代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <semaphore.h>
#define BUFFER_SIZE 10
// 缓冲区
int buffer[BUFFER_SIZE];
int in = 0, out = 0;
// 信号量
sem_t full, empty, mutex;
// 生产者线程函数
void *producer(void *arg) {
int item;
while (1) {
// 生产一个物品
item = rand() % 100;
// 等待缓冲区不满
sem_wait(&empty);
sem_wait(&mutex);
// 将物品放入缓冲区
buffer[in] = item;
in = (in + 1) % BUFFER_SIZE;
printf("Producer produced item %d\n", item);
sem_post(&mutex);
sem_post(&full);
}
}
// 消费者线程函数
void *consumer(void *arg) {
int item;
while (1) {
// 等待缓冲区不空
sem_wait(&full);
sem_wait(&mutex);
// 从缓冲区取出一个物品
item = buffer[out];
out = (out + 1) % BUFFER_SIZE;
printf("Consumer consumed item %d\n", item);
sem_post(&mutex);
sem_post(&empty);
}
}
int main() {
pthread_t prod, cons;
// 初始化信号量
sem_init(&mutex, 0, 1);
sem_init(&empty, 0, BUFFER_SIZE);
sem_init(&full, 0, 0);
// 创建生产者和消费者线程
pthread_create(&prod, NULL, producer, NULL);
pthread_create(&cons, NULL, consumer, NULL);
// 等待线程结束
pthread_join(prod, NULL);
pthread_join(cons, NULL);
// 销毁信号量
sem_destroy(&mutex);
sem_destroy(&empty);
sem_destroy(&full);
return 0;
}
```
在上面的代码中,使用了三个信号量:`full`表示缓冲区中物品的数量,`empty`表示缓冲区中空闲位置的数量,`mutex`用于互斥访问缓冲区。在生产者线程中,首先生产一个物品,然后等待`empty`信号量,表示缓冲区有空闲位置;同时等待`mutex`信号量,表示对缓冲区的访问是互斥的;将物品放入缓冲区后,释放`mutex`和`full`信号量。在消费者线程中,首先等待`full`信号量,表示缓冲区中有物品;同时等待`mutex`信号量,表示对缓冲区的访问是互斥的;从缓冲区中取出一个物品后,释放`mutex`和`empty`信号量。
注意:上面的代码只是示例,实际应用中需要注意信号量的初始化和销毁,以及线程的创建和销毁等问题。
阅读全文
相关推荐







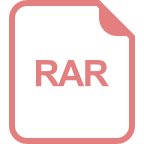








