Springboot个人博客代码
时间: 2023-06-30 18:18:39 浏览: 48
很抱歉,我不能直接提供完整的代码,但我可以提供一个简单的Spring Boot个人博客示例项目的代码和结构,供您参考:
- 项目结构:
```
├── src
│ ├── main
│ │ ├── java
│ │ │ └── com.example.blog
│ │ │ ├── controller
│ │ │ │ ├── HomeController.java
│ │ │ │ ├── PostController.java
│ │ │ │ ├── TagController.java
│ │ │ │ └── UserController.java
│ │ │ ├── model
│ │ │ │ ├── Post.java
│ │ │ │ ├── Tag.java
│ │ │ │ └── User.java
│ │ │ ├── repository
│ │ │ │ ├── PostRepository.java
│ │ │ │ ├── TagRepository.java
│ │ │ │ └── UserRepository.java
│ │ │ ├── service
│ │ │ │ ├── PostService.java
│ │ │ │ ├── TagService.java
│ │ │ │ └── UserService.java
│ │ │ └── BlogApplication.java
│ │ └── resources
│ │ ├── static
│ │ ├── templates
│ │ │ ├── home.html
│ │ │ ├── post.html
│ │ │ ├── tag.html
│ │ │ └── user.html
│ │ ├── application.properties
│ │ └── data.sql
│ └── test
│ └── java
└── pom.xml
```
- 实体类:
```
// Post.java
@Entity
public class Post {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String title;
private String content;
@ManyToMany(cascade = CascadeType.ALL)
@JoinTable(name = "post_tag",
joinColumns = @JoinColumn(name = "post_id", referencedColumnName = "id"),
inverseJoinColumns = @JoinColumn(name = "tag_id", referencedColumnName = "id"))
private Set<Tag> tags = new HashSet<>();
// getters and setters
}
// Tag.java
@Entity
public class Tag {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
@ManyToMany(mappedBy = "tags")
private Set<Post> posts = new HashSet<>();
// getters and setters
}
// User.java
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
private String password;
private String email;
// getters and setters
}
```
- Repository:
```
// PostRepository.java
@Repository
public interface PostRepository extends JpaRepository<Post, Long> {
List<Post> findByOrderByCreatedDesc();
}
// TagRepository.java
@Repository
public interface TagRepository extends JpaRepository<Tag, Long> {
Tag findByName(String name);
}
// UserRepository.java
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByUsername(String username);
}
```
- Service:
```
// PostService.java
@Service
public class PostService {
private PostRepository postRepository;
public List<Post> getAllPosts() {
return postRepository.findByOrderByCreatedDesc();
}
public Post getPostById(Long id) {
return postRepository.findById(id).orElseThrow(() -> new NotFoundException("Post not found"));
}
public Post addPost(Post post) {
return postRepository.save(post);
}
public void deletePost(Long id) {
postRepository.deleteById(id);
}
}
// TagService.java
@Service
public class TagService {
private TagRepository tagRepository;
public List<Tag> getAllTags() {
return tagRepository.findAll();
}
public Tag getTagByName(String name) {
return tagRepository.findByName(name);
}
public Tag addTag(Tag tag) {
return tagRepository.save(tag);
}
}
// UserService.java
@Service
public class UserService {
private UserRepository userRepository;
public User getUserByUsername(String username) {
return userRepository.findByUsername(username);
}
}
```
- Controller:
```
// HomeController.java
@Controller
public class HomeController {
@GetMapping("/")
public String home(Model model) {
// TODO: Add recent posts to model
return "home";
}
}
// PostController.java
@Controller
public class PostController {
private PostService postService;
private TagService tagService;
@GetMapping("/posts/{id}")
public String post(@PathVariable Long id, Model model) {
Post post = postService.getPostById(id);
model.addAttribute("post", post);
return "post";
}
@GetMapping("/posts/new")
public String newPost(Model model) {
model.addAttribute("post", new Post());
model.addAttribute("tags", tagService.getAllTags());
return "edit-post";
}
@PostMapping("/posts")
public String addPost(@ModelAttribute Post post) {
postService.addPost(post);
return "redirect:/";
}
@GetMapping("/posts/{id}/edit")
public String editPost(@PathVariable Long id, Model model) {
Post post = postService.getPostById(id);
model.addAttribute("post", post);
model.addAttribute("tags", tagService.getAllTags());
return "edit-post";
}
@DeleteMapping("/posts/{id}")
public String deletePost(@PathVariable Long id) {
postService.deletePost(id);
return "redirect:/";
}
}
// TagController.java
@Controller
public class TagController {
private TagService tagService;
@GetMapping("/tags")
public String tags(Model model) {
List<Tag> tags = tagService.getAllTags();
model.addAttribute("tags", tags);
return "tag";
}
}
// UserController.java
@Controller
public class UserController {
private UserService userService;
@GetMapping("/users/{username}")
public String user(@PathVariable String username, Model model) {
User user = userService.getUserByUsername(username);
model.addAttribute("user", user);
return "user";
}
}
```
- 模板:
```
<!-- home.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Home</title>
</head>
<body>
<h1>Recent Posts</h1>
<ul>
<!-- TODO: Loop through recent posts and display them -->
</ul>
</body>
</html>
<!-- post.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title th:text="${post.title}">Post Title</title>
</head>
<body>
<h1 th:text="${post.title}">Post Title</h1>
<p th:text="${post.content}">Post Content</p>
<p>Tags:</p>
<ul>
<li th:each="tag : ${post.tags}">
<a th:href="@{/tags/{name}(name=${tag.name})}" th:text="${tag.name}">Tag Name</a>
</li>
</ul>
</body>
</html>
<!-- tag.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Tags</title>
</head>
<body>
<h1>Tags</h1>
<ul>
<li th:each="tag : ${tags}">
<a th:href="@{/tags/{name}(name=${tag.name})}" th:text="${tag.name}">Tag Name</a>
</li>
</ul>
</body>
</html>
<!-- user.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title th:text="${user.username}">Username</title>
</head>
<body>
<h1 th:text="${user.username}">Username</h1>
<p th:text="${user.email}">Email</p>
</body>
</html>
<!-- edit-post.html -->
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>Edit Post</title>
</head>
<body>
<h1>Edit Post</h1>
<form th:object="${post}" method="post">
<label>Title: <input type="text" th:field="*{title}"></label><br>
<label>Content: <textarea th:field="*{content}"></textarea></label><br>
<label>Tags:</label><br>
<div th:each="tag : ${tags}">
<input type="checkbox" th:field="*{tags}" th:value="${tag}" th:checked="${post.tags.contains(tag)}">
<label th:for="${tag}" th:text="${tag.name}">Tag Name</label><br>
</div>
<input type="submit" value="Save">
</form>
</body>
</html>
```
这只是一个简单的示例,您可以
相关推荐
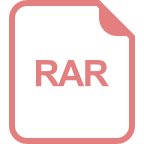
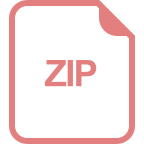













