java代码 RPC是一种远程过程调用技术,可以在不同的计算机之间调用远程方法。可以使用Java中的RMI(远程方法调用)或WebService等技术来实现此功能
时间: 2024-05-20 15:16:04 浏览: 64
下面是一个简单的Java RMI示例代码:
1.定义一个接口,包含需要远程调用的方法
public interface MyRemoteInterface extends Remote {
public String sayHello() throws RemoteException;
}
2.实现该接口的远程对象
public class MyRemoteObject implements MyRemoteInterface {
public String sayHello() throws RemoteException {
return "Hello, world!";
}
}
3.创建一个RMI注册表并将远程对象注册到该注册表中
public class MyRemoteServer {
public static void main(String[] args) {
try {
MyRemoteInterface remoteObj = new MyRemoteObject();
Naming.rebind("MyRemoteObj", remoteObj);
} catch (Exception e) {
e.printStackTrace();
}
}
}
4.创建一个RMI客户端程序,通过查找注册表并调用远程方法来调用远程对象
public class MyRemoteClient {
public static void main(String[] args) {
try {
MyRemoteInterface remoteObj = (MyRemoteInterface) Naming.lookup("MyRemoteObj");
String result = remoteObj.sayHello();
System.out.println(result);
} catch (Exception e) {
e.printStackTrace();
}
}
}
通过以上步骤,就可以在不同的计算机之间调用远程方法了。当客户端程序调用远程方法时,RMI会自动将参数和返回值进行序列化和反序列化,并将它们发送到远程对象上执行。
阅读全文
相关推荐


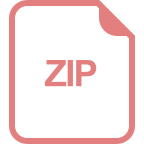
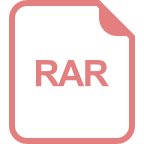
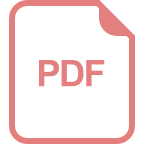
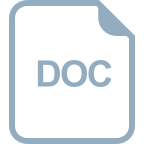
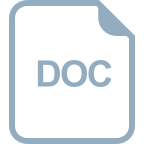
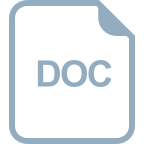
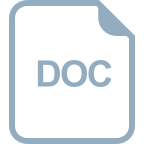
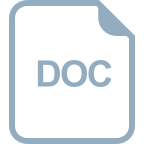
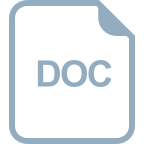
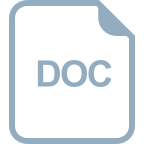
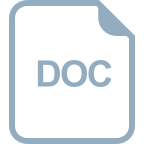
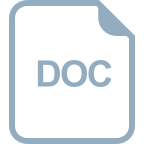
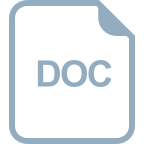
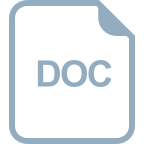