使用Python做一个界面,第一行是ABAQUS积分点数量:后面一个文本框用于输入数字,然后文本框后面写着积分点数量,同时将文本框里面输入的数字作为变量表示出来;最后一行有一个按钮,写着运行程序
时间: 2023-05-12 15:06:12 浏览: 265
可以使用Python的Tkinter模块来创建这个界面。以下是示例代码:
```python
import tkinter as tk
def run_program():
# 在这里编写运行程序的代码
pass
root = tk.Tk()
root.title("ABAQUS积分点数量")
label1 = tk.Label(root, text="ABAQUS积分点数量:")
label1.grid(row=0, column=0)
entry1 = tk.Entry(root)
entry1.grid(row=0, column=1)
label2 = tk.Label(root, text="积分点数量")
label2.grid(row=0, column=2)
button1 = tk.Button(root, text="运行程序", command=run_program)
button1.grid(row=1, column=1)
root.mainloop()
```
在这个界面中,我们创建了一个标签(label)来显示"ABAQUS积分点数量:",一个文本框(entry)用于输入数字,一个标签(label)来显示"积分点数量",以及一个按钮(button)用于运行程序。当用户点击按钮时,程序会调用run_program()函数来运行程序。你可以在这个函数中编写你的程序代码。
注意,这个示例代码中没有将文本框里面输入的数字作为变量表示出来,你需要自己添加这个功能。
相关问题
使用Python做一个界面,标题为软件接口,第一行Procast文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第二行ABAQUS文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第三行弹性模量文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第四行屈服强度文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第五行是ABAQUS积分点数量:后面一个文本框用于输入数字,然后文本框后面写着积分点数量;最后一行有一个按钮,写着运行程序。积分点数量做成一个变量,以便后面运行程序使用
以下是使用Python实现该界面的代码:
```python
import tkinter as tk
from tkinter import filedialog
class Interface:
def __init__(self, master):
self.master = master
master.title("软件接口")
# Procast文件
self.procast_label = tk.Label(master, text="Procast文件:")
self.procast_label.grid(row=0, column=0, sticky="w")
self.procast_text = tk.Text(master, height=1, width=50)
self.procast_text.grid(row=0, column=1)
self.procast_button = tk.Button(master, text="浏览", command=self.browse_procast)
self.procast_button.grid(row=0, column=2)
# ABAQUS文件
self.abaqus_label = tk.Label(master, text="ABAQUS文件:")
self.abaqus_label.grid(row=1, column=0, sticky="w")
self.abaqus_text = tk.Text(master, height=1, width=50)
self.abaqus_text.grid(row=1, column=1)
self.abaqus_button = tk.Button(master, text="浏览", command=self.browse_abaqus)
self.abaqus_button.grid(row=1, column=2)
# 弹性模量文件
self.elasticity_label = tk.Label(master, text="弹性模量文件:")
self.elasticity_label.grid(row=2, column=0, sticky="w")
self.elasticity_text = tk.Text(master, height=1, width=50)
self.elasticity_text.grid(row=2, column=1)
self.elasticity_button = tk.Button(master, text="浏览", command=self.browse_elasticity)
self.elasticity_button.grid(row=2, column=2)
# 屈服强度文件
self.yield_label = tk.Label(master, text="屈服强度文件:")
self.yield_label.grid(row=3, column=0, sticky="w")
self.yield_text = tk.Text(master, height=1, width=50)
self.yield_text.grid(row=3, column=1)
self.yield_button = tk.Button(master, text="浏览", command=self.browse_yield)
self.yield_button.grid(row=3, column=2)
# ABAQUS积分点数量
self.integral_label = tk.Label(master, text="ABAQUS积分点数量:")
self.integral_label.grid(row=4, column=0, sticky="w")
self.integral_entry = tk.Entry(master)
self.integral_entry.grid(row=4, column=1)
self.integral_text = tk.Label(master, text="积分点数量")
self.integral_text.grid(row=4, column=2)
# 运行程序按钮
self.run_button = tk.Button(master, text="运行程序", command=self.run_program)
self.run_button.grid(row=5, column=1)
def browse_procast(self):
filename = filedialog.askopenfilename()
self.procast_text.delete(1.0, tk.END)
self.procast_text.insert(tk.END, filename)
def browse_abaqus(self):
filename = filedialog.askopenfilename()
self.abaqus_text.delete(1.0, tk.END)
self.abaqus_text.insert(tk.END, filename)
def browse_elasticity(self):
filename = filedialog.askopenfilename()
self.elasticity_text.delete(1.0, tk.END)
self.elasticity_text.insert(tk.END, filename)
def browse_yield(self):
filename = filedialog.askopenfilename()
self.yield_text.delete(1.0, tk.END)
self.yield_text.insert(tk.END, filename)
def run_program(self):
procast_file = self.procast_text.get(1.0, tk.END).strip()
abaqus_file = self.abaqus_text.get(1.0, tk.END).strip()
elasticity_file = self.elasticity_text.get(1.0, tk.END).strip()
yield_file = self.yield_text.get(1.0, tk.END).strip()
integral_points = int(self.integral_entry.get())
# TODO: 运行程序
root = tk.Tk()
interface = Interface(root)
root.mainloop()
```
请注意,这只是一个简单的界面,需要根据实际需求进行修改和完善。
使用Python做一个界面,标题为软件接口,第一行Procast文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第二行ABAQUS文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第三行弹性模量文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第四行屈服强度文件:然后一个框用来记录之后浏览文件后的文字,最后是一个浏览文件;第五行是ABAQUS积分点数量:后面一个文本框用于输入数字,然后文本框后面写着积分点数量,将文本框里面的数字作为变量表示出来;最后一行有一个按钮,写着运行程序。
你可以使用 Python 的 tkinter 模块来创建这个界面。以下是一个简单的示例代码:
```python
import tkinter as tk
from tkinter import filedialog
# 创建窗口
root = tk.Tk()
root.title("软件接口")
# 创建 Procast 文件选择框
procast_label = tk.Label(root, text="Procast文件:")
procast_label.grid(row=0, column=0, sticky="w")
procast_text = tk.Text(root, height=1, width=50)
procast_text.grid(row=0, column=1)
def choose_procast_file():
filename = filedialog.askopenfilename()
procast_text.delete("1.0", "end")
procast_text.insert("end", filename)
procast_button = tk.Button(root, text="浏览", command=choose_procast_file)
procast_button.grid(row=0, column=2)
# 创建 ABAQUS 文件选择框
abaqus_label = tk.Label(root, text="ABAQUS文件:")
abaqus_label.grid(row=1, column=0, sticky="w")
abaqus_text = tk.Text(root, height=1, width=50)
abaqus_text.grid(row=1, column=1)
def choose_abaqus_file():
filename = filedialog.askopenfilename()
abaqus_text.delete("1.0", "end")
abaqus_text.insert("end", filename)
abaqus_button = tk.Button(root, text="浏览", command=choose_abaqus_file)
abaqus_button.grid(row=1, column=2)
# 创建弹性模量文件选择框
elastic_label = tk.Label(root, text="弹性模量文件:")
elastic_label.grid(row=2, column=0, sticky="w")
elastic_text = tk.Text(root, height=1, width=50)
elastic_text.grid(row=2, column=1)
def choose_elastic_file():
filename = filedialog.askopenfilename()
elastic_text.delete("1.0", "end")
elastic_text.insert("end", filename)
elastic_button = tk.Button(root, text="浏览", command=choose_elastic_file)
elastic_button.grid(row=2, column=2)
# 创建屈服强度文件选择框
yield_label = tk.Label(root, text="屈服强度文件:")
yield_label.grid(row=3, column=0, sticky="w")
yield_text = tk.Text(root, height=1, width=50)
yield_text.grid(row=3, column=1)
def choose_yield_file():
filename = filedialog.askopenfilename()
yield_text.delete("1.0", "end")
yield_text.insert("end", filename)
yield_button = tk.Button(root, text="浏览", command=choose_yield_file)
yield_button.grid(row=3, column=2)
# 创建 ABAQUS 积分点数量输入框
num_label = tk.Label(root, text="ABAQUS积分点数量:")
num_label.grid(row=4, column=0, sticky="w")
num_text = tk.Entry(root, width=10)
num_text.grid(row=4, column=1)
num_unit = tk.Label(root, text="积分点数量")
num_unit.grid(row=4, column=2)
# 创建运行程序按钮
def run_program():
# 在这里编写运行程序的代码
pass
run_button = tk.Button(root, text="运行程序", command=run_program)
run_button.grid(row=5, column=1)
# 运行窗口
root.mainloop()
```
这个界面包含了 4 个文件选择框和一个数字输入框,以及一个运行程序的按钮。你需要在 `run_program` 函数中编写运行程序的代码。
阅读全文
相关推荐
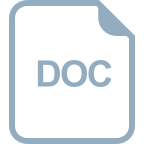
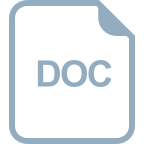
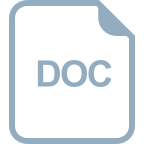
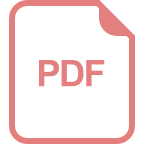
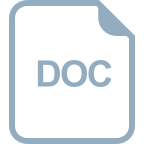
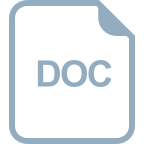
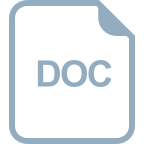


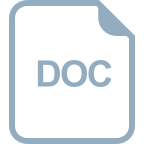
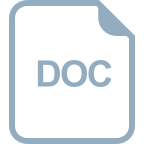
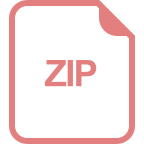
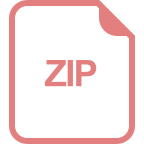
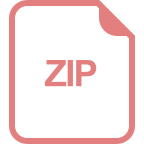
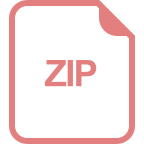
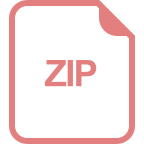