void writeOrder(string filename, const Order& order) { ofstream out(filename, ios::app); out << "订单号:" << order.getOrderId() << endl; out << "时间:" << ctime(&order.getTimeOfOrder()); out << "客户信息:" << order.getCustomer().getName() << " " << order.getCustomer().getAccount() << endl; out << "书本信息:" << endl; for (const auto& book : order.getBooks()) { out << book.getTitle() << " " << book.getAuthor() << " " << book.getDescription() << " " << book.getPrice() << endl; } out << "总价:" << order.getTotalPrice() << endl; out << endl; out.close(); }改错
时间: 2023-10-30 13:14:25 浏览: 33
这段代码看上去没有明显的错误,但是有一些可以改进的地方:
1. 文件名应该使用 const std::string& 类型的引用传递,这样可以避免不必要的拷贝操作;
2. getOrderId() 和 getTimeOfOrder() 的返回值应该是 const 类型的,因为这些方法不应该修改订单对象的状态;
3. ctime() 函数返回的是一个 C 风格字符串,需要使用 std::string() 将其转换成 std::string 类型的字符串;
4. 在写入书本信息时,应该添加一些分隔符,以便于在读取时进行分割;
5. 在写入文件时,应该使用异常处理机制,以便于在出错时能够及时捕获异常并进行处理。
改进后的代码如下所示:
```c++
void writeOrder(const std::string& filename, const Order& order) {
try {
std::ofstream out(filename, std::ios::app);
if (!out.is_open()) {
throw std::runtime_error("无法打开文件 " + filename);
}
out << "订单号:" << order.getOrderId() << std::endl;
out << "时间:" << std::string(ctime(&order.getTimeOfOrder()));
out << "客户信息:" << order.getCustomer().getName() << " " << order.getCustomer().getAccount() << std::endl;
out << "书本信息:" << std::endl;
for (const auto& book : order.getBooks()) {
out << book.getTitle() << "|" << book.getAuthor() << "|" << book.getDescription() << "|" << book.getPrice() << std::endl;
}
out << "总价:" << order.getTotalPrice() << std::endl;
out << std::endl;
out.close();
}
catch (const std::exception& ex) {
std::cerr << ex.what() << std::endl;
}
}
```
改进后的代码使用了 try-catch 语句进行异常处理,如果无法打开文件,则会抛出一个 std::runtime_error 异常,并将异常信息输出到标准错误流中。在写入书本信息时,使用了竖杠(|)作为分隔符,方便在读取时进行分割。
相关推荐
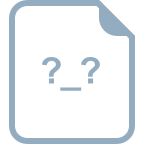
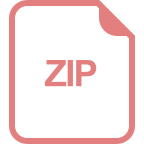
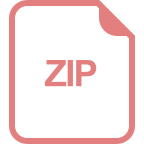














