router.beforeEach(async (_to, _from, next) => { if (_from.path === '/answer-complete' && _to.path === '/answer-card') { // 这里让成绩页不能返回答题卡 next({ path: '/answer-mode', replace: true }) } else if (_from.path === '/create-note') { showDialog({ title: '退出笔记', message: '笔记还未保存,确定丢弃吗?', confirmButtonText: '保存并退出', confirmButtonColor: '#2E6EF4', cancelButtonText: '直接退出', cancelButtonColor: '#666' }).then(() => { next() }).catch(() => { next(false) }); } else next(); });这段代码,为什么从creaete-note页面返回时,不弹窗
时间: 2024-04-06 22:31:52 浏览: 81
这段代码中,当从`create-note`页面返回时,应该会弹出提示窗口,但是具体原因需要根据代码环境进行分析。以下是一些可能导致问题的原因:
1. 在`showDialog`方法中,`confirmButtonText`和`cancelButtonText`的值可能为空或未正确设置,导致无法显示按钮。
2. `showDialog`方法可能未正确引入或未正确封装,导致无法弹出提示窗口。
3. `create-note`页面可能未正确绑定`beforeRouteLeave`守卫,导致页面返回时无法触发拦截。
4. `create-note`页面中的内容可能已经被保存,导致无法触发拦截。
你可以仔细检查以上几个方面,看看是不是其中的一个原因导致了弹窗无法弹出的问题。另外,你也可以在`create-note`页面中添加打印语句,查看该页面是否正确触发了`beforeRouteLeave`守卫。
相关问题
router.beforeEach(async (_to, _from, next) => { console.log(_from); if (_from.path === '/answer-complete' && _to.path === '/answer-card') { // 这里让成绩页不能返回答题卡 next({ path: '/answer-mode', replace: true }) } else if (_from.path === '/create-note') { console.log(222); await showConfirmDialog({ title: '退出笔记', message: '笔记还未保存,确定丢弃吗?', confirmButtonText: '保存并退出', confirmButtonColor: '#2E6EF4', cancelButtonText: '直接退出', cancelButtonColor: '#666' }).then(() => { next() }).catch(() => { next() }); } else next(); });在这段代码中,我从create-note页面保存退出后,再进去,再退出时,没有弹出模态框
根据你提供的代码,这段逻辑应该是在路由导航守卫中,其中 `beforeEach` 函数会在每次路由跳转前被调用。从 `create-note` 页面退出时,会弹出模态框询问是否保存并退出,但是从 `create-note` 再次进入,再退出时没有弹出模态框。
我猜测可能是因为在第一次离开 `create-note` 页面时,由于异步操作 `showConfirmDialog` 的存在,导致 `next()` 函数被提前调用了,路由被立即跳转到了其他页面,而模态框并没有完全弹出。当你再次进入 `create-note` 页面时,路由已经被跳转到了其他页面,因此再次退出时就没有弹出模态框了。
为了解决这个问题,你可以试着在 `showConfirmDialog` 的 `then` 和 `catch` 回调函数中调用 `next()` 函数,而不是在 `beforeEach` 函数中调用。这样可以确保模态框完全弹出后再进行路由跳转。具体代码如下:
```
router.beforeEach(async (_to, _from, next) => {
console.log(_from);
if (_from.path === '/answer-complete' && _to.path === '/answer-card') {
// 这里让成绩页不能返回答题卡
next({ path: '/answer-mode', replace: true })
} else if (_from.path === '/create-note') {
console.log(222);
await showConfirmDialog({
title: '退出笔记',
message: '笔记还未保存,确定丢弃吗?',
confirmButtonText: '保存并退出',
confirmButtonColor: '#2E6EF4',
cancelButtonText: '直接退出',
cancelButtonColor: '#666'
}).then(() => {
next(); // 在这里调用 next() 函数
}).catch(() => {
next(); // 在这里调用 next() 函数
});
} else {
next();
}
});
```
希望这个解答能够帮助到你!
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter) import Home from './components/HelloWorld.vue' var router = new VueRouter({ // 创建路由实例对象router routes: [ { path: '/', redirect: '/home', meta: { title: '首页' } }, { path: '/home', component: Home, name: 'home', meta: { title: '首页' } }, ] ,router.beforeEach((to, from, next) => { if (to.meta.title) { document.title = to.meta.title }; next(); }) }) export default router 报错
你的代码中,`router.beforeEach()` 方法应该放在 `routes` 数组后面,而不是放在外面,因为 `router` 对象是在 `routes` 中创建的。也就是说,将 `router.beforeEach()` 方法移动到 `routes` 数组后面即可,如下所示:
```
import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter)
import Home from './components/HelloWorld.vue'
var router = new VueRouter({
// 创建路由实例对象router
routes: [
{ path: '/', redirect: '/home', meta: { title: '首页' } },
{ path: '/home', component: Home, name: 'home', meta: { title: '首页' } },
]
})
router.beforeEach((to, from, next) => {
if (to.meta.title) {
document.title = to.meta.title
};
next();
})
export default router
```
这样应该就可以解决报错问题了。
阅读全文
相关推荐
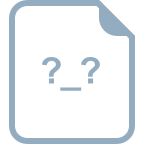
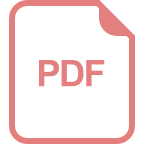
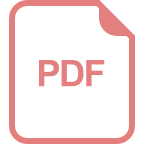
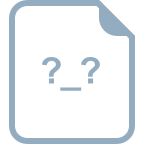

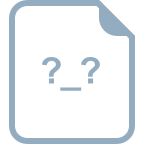
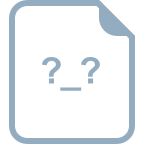
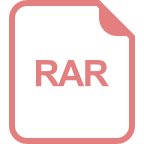
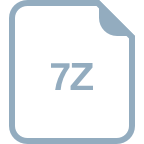
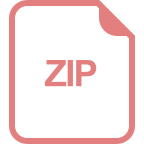
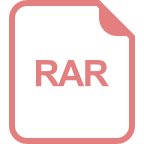
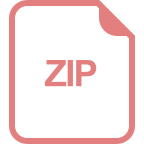
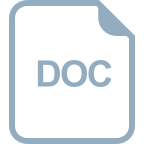
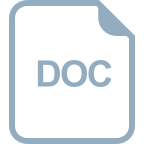