python统计单词个数
时间: 2023-06-20 09:09:16 浏览: 1574
可以使用Python中的字典来统计单词个数,具体步骤如下:
1. 读取文本内容
2. 将文本内容按照空格分割成单词列表
3. 遍历单词列表,将每个单词作为字典的键,出现次数作为值存入字典中
4. 输出字典中各个单词的出现次数
示例代码如下:
```python
# 读取文本内容
with open('text.txt', 'r') as file:
content = file.read()
# 将文本内容按照空格分割成单词列表
words = content.split()
# 统计单词个数
word_count = {}
for word in words:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
# 输出字典中各个单词的出现次数
for word, count in word_count.items():
print(word, count)
```
其中`text.txt`是待统计单词的文本文件名,可以根据需要修改。
相关问题
用Python统计单词个数
好的,我能够回答这个问题。统计单词个数可以使用Python中的一些内置函数和模块来实现。具体实现方法可以参考如下代码:
```python
# 打开文件
with open('file.txt', 'r') as f:
# 读取文件中的内容
text = f.read()
# 统计单词个数
words = text.split()
word_count = len(words)
# 输出单词个数
print(word_count)
```
其中,`file.txt`是待统计单词个数的文本文件名。程序首先打开文件,然后读取其中的内容,使用`split()`方法将文本分隔成单个的单词,最后使用`len()`函数统计单词的个数并输出。
python统计单词个数并输出最长单词
可以使用Python的collections模块中的Counter函数来统计单词个数,然后使用max函数找到最长的单词。以下是示例代码:
```python
from collections import Counter
text = "This is a sample text with some words. This is another sentence with more words."
# 统计单词个数
word_counts = Counter(text.split())
# 输出单词个数
print("单词个数:", sum(word_counts.values()))
# 输出最长单词
longest_word = max(word_counts, key=len)
print("最长单词:", longest_word)
```
输出结果为:
```
单词个数: 15
最长单词: sentence
```
阅读全文
相关推荐
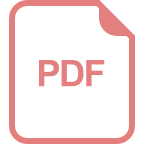






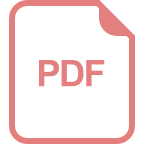
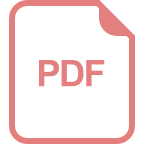
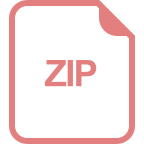