C++Write a program to achieve the following requirements: 1) The Square class is defined. Its properties are (protection type): side length, area function (area ()) and constructor are provided; 2) Rectangle class is derived from square class, and its properties include: length and width, area () function and constructor; 3) Write a main function, initialize objects defined by Square class and Rectangle class, and output their area respectively.
时间: 2024-03-15 07:46:10 浏览: 82
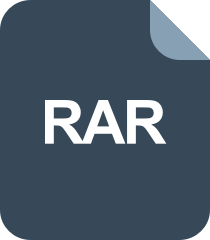
C++how to program
Sure, here's a C++ program that meets your requirements:
```c++
#include <iostream>
using namespace std;
class Square {
protected:
float side;
public:
Square(float s) {
side = s;
}
float area() {
return side * side;
}
};
class Rectangle : public Square {
protected:
float length, width;
public:
Rectangle(float l, float w) : Square(l) {
length = l;
width = w;
}
float area() {
return length * width;
}
};
int main() {
Square sq(5.0);
Rectangle rec(4.0, 6.0);
cout << "Area of square: " << sq.area() << endl;
cout << "Area of rectangle: " << rec.area() << endl;
return 0;
}
```
In this program, we define a `Square` class with a `side` property, an `area()` function, and a constructor that takes the `side` value as a parameter. We also define a `Rectangle` class that inherits from `Square` and adds `length` and `width` properties and its own `area()` function.
In the `main()` function, we create an object of the `Square` class with a side length of 5.0 and an object of the `Rectangle` class with a length of 4.0 and a width of 6.0. We then call the `area()` function for each object and output the results.
The output of the program should be:
```
Area of square: 25
Area of rectangle: 24
```
阅读全文
相关推荐
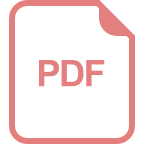
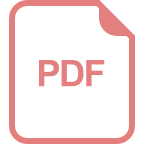
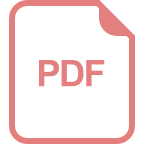
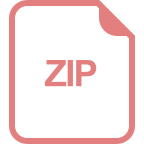
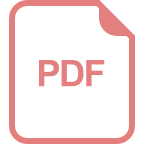
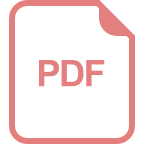
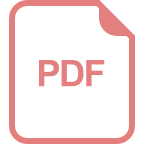
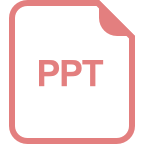
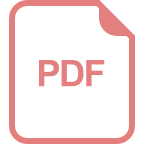
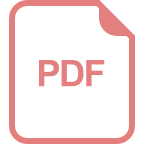
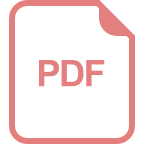
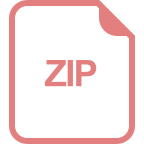
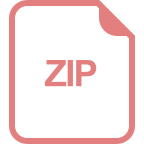