RecyclerView只要前十条数据
时间: 2023-11-16 13:24:07 浏览: 49
你可以通过设置RecyclerView的LayoutManager来实现只显示前十条数据。具体来说,你可以使用LinearLayoutManager,并调用setInitialPrefetchItemCount方法将其初始化预取项数目设置为10。这样,RecyclerView在初始化时就只会加载前10个数据项,而不会加载整个数据集。另外,你也可以在Adapter中的getItemCount方法中返回10,这样RecyclerView也只会显示前10条数据。
相关问题
如何查看前十条数据
要查看前十条数据,也需要知道数据的具体形式和编程语言。一般情况下,我们可以通过以下方式查看前十条数据。
在Python中,假设数据存储在一个列表或者数组中,可以通过以下方式查看前十条数据:
```
print(data[:10]) # 切片操作,取前10条数据
```
在Java中,假设数据存储在一个数组中,可以通过以下方式查看前十条数据:
```
for(int i = 0; i < 10; i++) {
System.out.println(arr[i]);
}
```
在C++中,假设数据存储在一个数组中,可以通过以下方式查看前十条数据:
```
for(int i = 0; i < 10; i++) {
cout << arr[i] << endl;
}
```
以上仅是一些示例,具体实现方式还需要根据具体情况而定。
pymongo删除前十条数据
假设你已经连接到 MongoDB 数据库并选中了相应的集合,可以使用以下代码删除前十条数据:
```python
import pymongo
# 连接到 MongoDB
client = pymongo.MongoClient("mongodb://localhost:27017/")
# 选择数据库和集合
db = client["mydatabase"]
collection = db["mycollection"]
# 删除前十条数据
result = collection.delete_many({}).deleted_count
print(f"已删除 {result} 条数据")
```
在上述代码中,我们使用了 `delete_many` 方法来删除所有数据,并使用 `deleted_count` 属性获取已删除的文档数量。
如果你只想删除前十条数据,可以在 `delete_many` 方法中添加一个查询条件,例如:
```python
result = collection.delete_many({}).limit(10).deleted_count
```
在上述代码中,我们添加了 `limit(10)` 条件,表示只删除前十条数据。
相关推荐
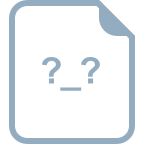
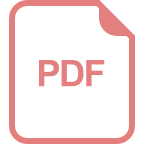
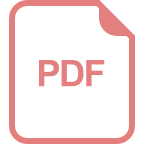












