基于机器学习的无线通信信号质量评估实验代码
时间: 2024-09-06 17:03:39 浏览: 29
在基于机器学习的无线通信信号质量评估实验中,通常会涉及到信号的采集、预处理、特征提取、模型训练和评估等步骤。以下是一个简化的代码示例,使用Python语言和一些常用的库来实现这样一个实验:
```python
import numpy as np
import pandas as pd
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
from sklearn.metrics import classification_report
from scipy import signal
# 假设我们已经有了一些信号数据和对应的质量标签,这里用随机数据模拟
# 实际中应该是通过无线通信设备采集的信号数据
np.random.seed(0)
data = np.random.rand(1000, 100) # 假设有1000个样本,每个样本有100个特征
labels = np.random.randint(0, 2, 1000) # 假设有两个质量等级:0和1
# 将数据集分为训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(data, labels, test_size=0.2, random_state=42)
# 使用随机森林分类器作为评估模型
clf = RandomForestClassifier(n_estimators=100)
# 训练模型
clf.fit(X_train, y_train)
# 预测测试集结果
y_pred = clf.predict(X_test)
# 评估模型
report = classification_report(y_test, y_pred)
print(report)
# 如果需要使用特定的无线信号特征,可以在这里进行特征提取
# 例如,可以从信号中提取功率、信噪比、误码率等特征
# 下面是一个简单的例子,使用功率谱密度作为特征
def extract_features(signal_data):
freqs, psd = signal.welch(signal_data, nperseg=128)
return np.mean(psd)
# 提取特征
features = np.array([extract_features(sig) for sig in data])
# 重新划分数据集,这里省略了特征提取后的数据处理步骤
# X_train_features, X_test_features, y_train, y_test = ...
# 重新训练模型并评估
# clf.fit(X_train_features, y_train)
# y_pred = clf.predict(X_test_features)
# report = classification_report(y_test, y_pred)
# print(report)
```
请注意,这个代码只是一个非常简化的示例,实际应用中需要根据具体的信号数据和问题来设计特征提取方法和选择合适的机器学习模型。此外,还需要对数据进行详细的预处理,以确保模型可以从中学习到有效的信息。
阅读全文
相关推荐
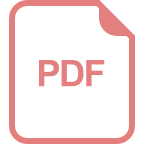
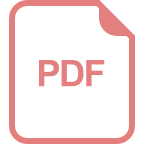
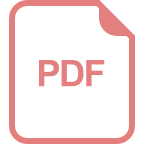
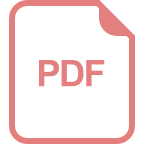
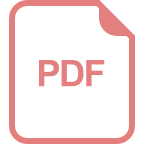
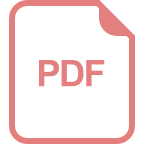
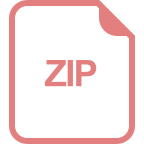
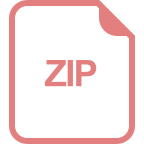
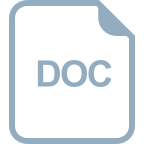
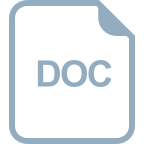
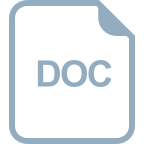
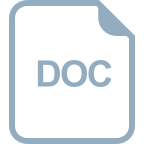
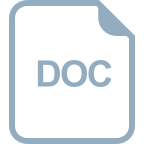
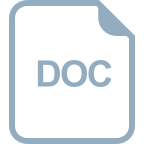
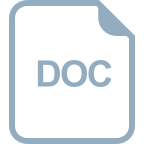
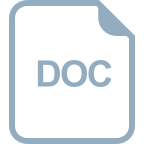
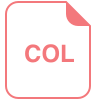
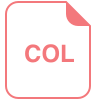
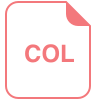