用python和MySQL写一个食堂查询系统,并由登录页面,页面可以为web页面
时间: 2024-05-16 17:18:24 浏览: 134
好的,下面是一个简单的食堂查询系统的Python代码,使用MySQL作为数据库,并使用Flask作为Web框架:
1. 安装必要的包
```python
pip install Flask flask-mysqldb
```
2. 创建数据库表
在MySQL中创建一个名为`canteen`的数据库,并执行以下SQL语句创建`users`和`meals`表:
```sql
CREATE TABLE users (
id INT(11) AUTO_INCREMENT PRIMARY KEY,
username VARCHAR(50) NOT NULL UNIQUE,
password VARCHAR(100) NOT NULL
);
CREATE TABLE meals (
id INT(11) AUTO_INCREMENT PRIMARY KEY,
name VARCHAR(100) NOT NULL,
price DECIMAL(5,2) NOT NULL,
description VARCHAR(500),
image_url VARCHAR(500)
);
```
3. 编写Python代码
```python
from flask import Flask, render_template, request, redirect, url_for, session
from flask_mysqldb import MySQL
import bcrypt
app = Flask(__name__)
app.secret_key = 'secret'
app.config['MYSQL_HOST'] = 'localhost'
app.config['MYSQL_USER'] = 'root'
app.config['MYSQL_PASSWORD'] = 'password'
app.config['MYSQL_DB'] = 'canteen'
mysql = MySQL(app)
@app.route('/')
def index():
cur = mysql.connection.cursor()
cur.execute('SELECT * FROM meals')
meals = cur.fetchall()
cur.close()
return render_template('index.html', meals=meals)
@app.route('/register', methods=['GET', 'POST'])
def register():
if request.method == 'POST':
username = request.form['username']
password = bcrypt.hashpw(request.form['password'].encode('utf-8'), bcrypt.gensalt())
cur = mysql.connection.cursor()
cur.execute('INSERT INTO users (username, password) VALUES (%s, %s)', (username, password))
mysql.connection.commit()
cur.close()
session['username'] = username
return redirect(url_for('index'))
else:
return render_template('register.html')
@app.route('/login', methods=['GET', 'POST'])
def login():
if request.method == 'POST':
username = request.form['username']
password = request.form['password'].encode('utf-8')
cur = mysql.connection.cursor()
cur.execute('SELECT * FROM users WHERE username = %s', [username])
user = cur.fetchone()
cur.close()
if user and bcrypt.checkpw(password, user['password'].encode('utf-8')):
session['username'] = username
return redirect(url_for('index'))
else:
error = 'Invalid username or password'
return render_template('login.html', error=error)
else:
return render_template('login.html')
@app.route('/logout')
def logout():
session.pop('username', None)
return redirect(url_for('index'))
@app.route('/add', methods=['GET', 'POST'])
def add():
if 'username' not in session:
return redirect(url_for('login'))
if request.method == 'POST':
name = request.form['name']
price = request.form['price']
description = request.form['description']
image_url = request.form['image_url']
cur = mysql.connection.cursor()
cur.execute('INSERT INTO meals (name, price, description, image_url) VALUES (%s, %s, %s, %s)', (name, price, description, image_url))
mysql.connection.commit()
cur.close()
return redirect(url_for('index'))
else:
return render_template('add.html')
if __name__ == '__main__':
app.run(debug=True)
```
4. 创建HTML模板
在项目根目录下创建一个名为`templates`的文件夹,并在其中创建以下HTML模板:
index.html:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Canteen</title>
</head>
<body>
{% if 'username' in session %}
<p>Welcome, {{ session['username'] }}!</p>
<a href="{{ url_for('add') }}">Add meal</a>
<a href="{{ url_for('logout') }}">Logout</a>
{% else %}
<a href="{{ url_for('login') }}">Login</a>
<a href="{{ url_for('register') }}">Register</a>
{% endif %}
<hr>
{% for meal in meals %}
<h2>{{ meal['name'] }}</h2>
<p>${{ meal['price'] }}</p>
<p>{{ meal['description'] }}</p>
<img src="{{ meal['image_url'] }}" alt="{{ meal['name'] }}">
{% endfor %}
</body>
</html>
```
register.html:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Register</title>
</head>
<body>
<h1>Register</h1>
{% if error %}
<p style="color: red;">{{ error }}</p>
{% endif %}
<form method="post">
<label>Username:</label>
<input type="text" name="username" required>
<br>
<label>Password:</label>
<input type="password" name="password" required>
<br>
<input type="submit" value="Register">
</form>
</body>
</html>
```
login.html:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login</title>
</head>
<body>
<h1>Login</h1>
{% if error %}
<p style="color: red;">{{ error }}</p>
{% endif %}
<form method="post">
<label>Username:</label>
<input type="text" name="username" required>
<br>
<label>Password:</label>
<input type="password" name="password" required>
<br>
<input type="submit" value="Login">
</form>
</body>
</html>
```
add.html:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Add meal</title>
</head>
<body>
<h1>Add meal</h1>
<form method="post">
<label>Name:</label>
<input type="text" name="name" required>
<br>
<label>Price:</label>
<input type="number" name="price" step="0.01" min="0" required>
<br>
<label>Description:</label>
<textarea name="description"></textarea>
<br>
<label>Image URL:</label>
<input type="text" name="image_url">
<br>
<input type="submit" value="Add">
</form>
</body>
</html>
```
5. 运行Web应用程序
在命令行中运行以下命令启动Web应用程序:
```python
python app.py
```
然后在浏览器中输入`http://localhost:5000`查看应用程序。
阅读全文
相关推荐
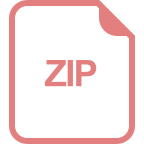

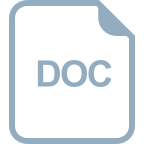
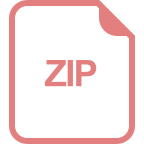
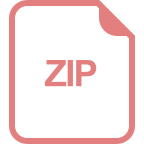
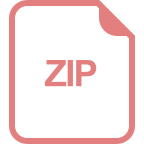
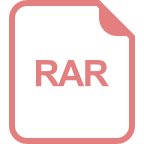
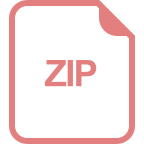
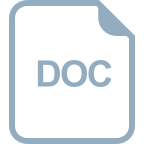
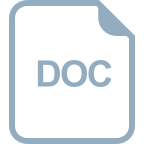
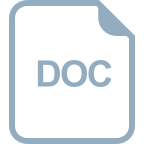
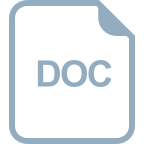
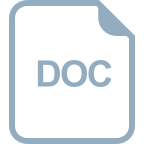
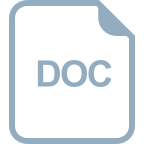
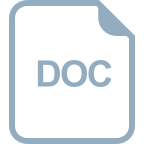
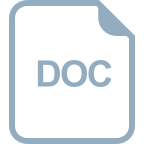
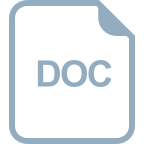