public void test01(){ ApplicationContext context= new ClassPathXmlApplicationContext("application-context.xml"); Student student =(Student) context.getBean("student"); StudentService studentService = context.getBean("studentService", StudentService.class); /*添加学生信息*/ studentService.addStudent(student); /*通过学生id查询学生信息*/ Student student1 = studentService.selectStudentByID(1); System.out.println(student1); /*通过班级id查询学生信息*/ List<Student> liststudent = studentService.selectStudentByCID(1); for (Student s:liststudent) { System.out.println(s); } /*任务10: * 修改学生信息【5分】 * 删除学生信息【5分】*/ } }
时间: 2024-02-14 11:15:02 浏览: 65
这段代码是一个测试方法,主要测试了使用 Spring 框架进行依赖注入和调用服务层方法的流程,其中包括添加学生信息、通过学生id查询学生信息、通过班级id查询学生信息。
下面给出修改和删除学生信息的代码:
修改学生信息:
```java
// 根据学生id获取需要修改的学生信息
Student student = studentService.selectStudentByID(id);
// 设置需要修改的属性值
student.setName(name);
student.setAge(age);
student.setGender(gender);
student.setMajor(major);
// 更新学生信息
studentService.updateStudent(student);
```
删除学生信息:
```java
// 根据学生id删除学生信息
studentService.deleteStudent(id);
```
相关问题
package jdbc.score.test; import java.util.List; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import jdbc.score.dao.impl.CourseDaoImpl; import jdbc.score.entities.Course; public class TestCourse { public static void main(String[] args) { // TODO Auto-generated method stub ApplicationContext applicationContext=new ClassPathXmlApplicationContext("spring.jdbc.xml"); //调用CourseDaoImpl类的对应方法查询选课模式为“专业”的课程,并显示它们的信息 .............(代码2) } }
在这段代码中,代码2处应该调用CourseDaoImpl类的对应方法查询选课模式为“专业”的课程,并显示它们的信息。可以使用以下代码实现:
```java
CourseDaoImpl courseDaoImpl = applicationContext.getBean("courseDaoImpl", CourseDaoImpl.class);
List<Course> courses = courseDaoImpl.getCoursesByMode("专业");
for (Course course : courses) {
System.out.println(course);
}
```
其中,`getBean()`方法用于获取Spring容器中的CourseDaoImpl对象,`getCoursesByMode()`方法是CourseDaoImpl类中用于查询选课模式为“专业”的课程的方法,返回一个包含Course对象的List集合,最后通过循环输出每个课程的信息。
package com.phonemarket.listener; import java.util.List; import javax.servlet.ServletContext; import javax.servlet.ServletContextEvent; import javax.servlet.ServletContextListener; import javax.servlet.annotation.WebListener; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; import com.phonemarket.entity.Banner; import com.phonemarket.service.IBannerService; /** * @author 张贤 * */ public class BannerListener implements ServletContextListener { @Override public void contextDestroyed(ServletContextEvent arg0) { // TODO Auto-generated method stub } @Override public void contextInitialized(ServletContextEvent arg0) { ApplicationContext app=new ClassPathXmlApplicationContext("applicationContext.xml"); IBannerService bannerService = app.getBean(IBannerService.class); ServletContext application = arg0.getServletContext(); List<Banner> bannerList = bannerService.findAllShowBanner(); application.setAttribute("bannerList", bannerList); } }
这是一个实现了ServletContextListener接口的BannerListener类,用于监听Web应用程序的上下文初始化和销毁事件。在上下文初始化时,该类会从Spring容器中获取一个IBannerService实例,并调用其findAllShowBanner()方法获取所有需要展示的轮播图列表,并将其存储在ServletContext中,以便在整个应用程序中可以访问这些轮播图信息。这个类可能是在Web应用程序中使用的一个监听器,用于在应用程序启动时加载一些数据等操作。
阅读全文
相关推荐
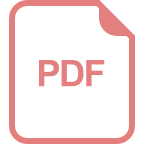
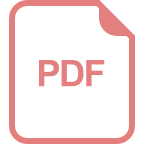
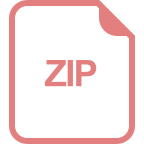


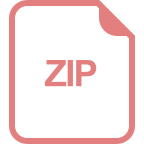
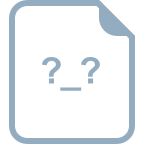
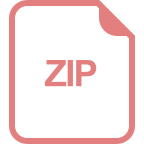
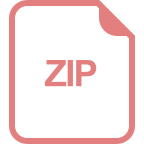
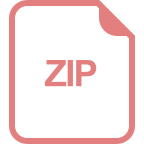
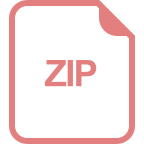
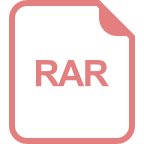