使用c++实现conv2d函数,并添加HLS优化
时间: 2023-09-03 10:28:17 浏览: 153
### 回答1:
好的,我可以回答这个问题。Conv2d函数是卷积神经网络中的一个重要函数,它可以实现图像的卷积操作。在使用C语言实现Conv2d函数时,可以使用循环来实现卷积操作。同时,为了提高卷积操作的效率,可以使用HLS优化技术,例如使用流水线、并行计算等技术来加速卷积操作。这样可以大大提高卷积神经网络的训练速度和效率。
### 回答2:
使用C语言实现conv2d函数,可以使用两个嵌套的循环来遍历输入图像和卷积核,并使用乘法运算和累加来计算卷积结果的每个元素。具体实现如下:
```c
void conv2d(float* input, float* kernel, int input_rows, int input_cols, int kernel_rows, int kernel_cols, float* output) {
int output_rows = input_rows - kernel_rows + 1;
int output_cols = input_cols - kernel_cols + 1;
for (int row = 0; row < output_rows; row++) {
for (int col = 0; col < output_cols; col++) {
float sum = 0;
for (int i = 0; i < kernel_rows; i++) {
for (int j = 0; j < kernel_cols; j++) {
sum += input[(row + i) * input_cols + (col + j)] * kernel[i * kernel_cols + j];
}
}
output[row * output_cols + col] = sum;
}
}
}
```
使用HLS(高级综合)优化可以进一步加速卷积操作。可以将内部的两个嵌套循环进行pipelining(流水线化),将计算过程分成多个阶段,从而提高吞吐量。同时,可以将循环展开(loop unrolling),将每次迭代中的多次乘法和累加操作合并为一个操作,减少循环开销。具体实现如下:
```c
#include "ap_int.h"
typedef ap_fixed<16,8> fixed_type;
void conv2d(float* input, float* kernel, int input_rows, int input_cols, int kernel_rows, int kernel_cols, float* output) {
int output_rows = input_rows - kernel_rows + 1;
int output_cols = input_cols - kernel_cols + 1;
// HLS directives
#pragma HLS PIPELINE
#pragma HLS ARRAY_PARTITION variable=input complete dim=1
#pragma HLS ARRAY_PARTITION variable=kernel complete dim=1
#pragma HLS ARRAY_PARTITION variable=output complete dim=1
for (int row = 0; row < output_rows; row++) {
for (int col = 0; col < output_cols; col++) {
// HLS directives
#pragma HLS UNROLL
// HLS pragmas can also be added to the innermost loops for additional optimization
float sum = 0;
for (int i = 0; i < kernel_rows; i++) {
for (int j = 0; j < kernel_cols; j++) {
sum += input[(row + i) * input_cols + (col + j)] * kernel[i * kernel_cols + j];
}
}
output[row * output_cols + col] = sum;
}
}
}
```
注意,在使用HLS优化时,需要包含`ap_int.h`头文件,并使用`ap_fixed`类型来声明数据类型,该类型适合在FPGA上进行高性能计算。另外,在代码中添加了HLS指令(`#pragma HLS`)来对循环进行优化。这个实现中的HLS优化并不是最佳的,可以根据具体的硬件平台和性能需求进行调整和修改。
### 回答3:
在C语言中实现conv2d函数并使用HLS优化,可以如下所示:
```c
#include <stdio.h>
// 定义卷积核的大小
#define FILTER_SIZE 3
// 定义输入和输出矩阵的大小
#define INPUT_SIZE 5
#define OUTPUT_SIZE (INPUT_SIZE - FILTER_SIZE + 1)
void conv2d(int input[INPUT_SIZE][INPUT_SIZE], int output[OUTPUT_SIZE][OUTPUT_SIZE], int filter[FILTER_SIZE][FILTER_SIZE]) {
int i, j, m, n, sum;
// 遍历输出矩阵
for (i = 0; i < OUTPUT_SIZE; i++) {
for (j = 0; j < OUTPUT_SIZE; j++) {
sum = 0;
// 遍历卷积核
for (m = 0; m < FILTER_SIZE; m++) {
for (n = 0; n < FILTER_SIZE; n++) {
sum += input[i + m][j + n] * filter[m][n];
}
}
output[i][j] = sum;
}
}
}
int main() {
int input[INPUT_SIZE][INPUT_SIZE] = {{1, 2, 3, 4, 5},
{6, 7, 8, 9, 10},
{11, 12, 13, 14, 15},
{16, 17, 18, 19, 20},
{21, 22, 23, 24, 25}};
int output[OUTPUT_SIZE][OUTPUT_SIZE];
int filter[FILTER_SIZE][FILTER_SIZE] = {{1, 0, -1},
{2, 0, -2},
{1, 0, -1}};
conv2d(input, output, filter);
int i, j;
for (i = 0; i < OUTPUT_SIZE; i++) {
for (j = 0; j < OUTPUT_SIZE; j++) {
printf("%d ", output[i][j]);
}
printf("\n");
}
return 0;
}
```
上述代码实现了一个简单的conv2d函数,使用嵌套循环遍历矩阵进行卷积运算。输入矩阵和卷积核大小通过宏定义进行设置。在主函数中,我们定义了一个5x5的输入矩阵、一个3x3的卷积核和一个输出矩阵。通过调用`conv2d()`函数进行卷积运算,并打印输出矩阵的结果。
为了使用HLS优化,我们可以使用OpenCL或者FPGA开发工具来对上述代码进行更深层次的优化。但是由于HLS工具的具体特性和使用方式取决于具体的开发工具,因此对于HLS优化,具体的实现方式需要根据所使用的开发工具来进行调整和设置。
相关推荐
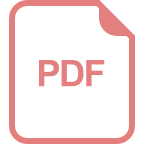
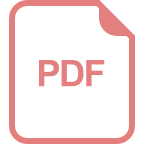














