stm32f103c8t6串口1数据收发代码
时间: 2023-08-03 18:02:44 浏览: 107
下面是一个基本的 STM32F103C8T6(也称为“蓝板”)上使用串口1进行数据收发的示例代码:
```c
#include "stm32f10x.h"
void USART1_Init(void) {
GPIO_InitTypeDef GPIO_InitStruct;
USART_InitTypeDef USART_InitStruct;
// 使能串口1和GPIOA的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_USART1 | RCC_APB2Periph_GPIOA, ENABLE);
// 配置USART1引脚
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_9; // USART1 Tx (PA9)
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_AF_PP; // 复用推挽输出
GPIO_InitStruct.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStruct);
GPIO_InitStruct.GPIO_Pin = GPIO_Pin_10; // USART1 Rx (PA10)
GPIO_InitStruct.GPIO_Mode = GPIO_Mode_IN_FLOATING; // 浮空输入
GPIO_Init(GPIOA, &GPIO_InitStruct);
// 配置USART1参数
USART_InitStruct.USART_BaudRate = 9600; // 波特率为9600
USART_InitStruct.USART_WordLength = USART_WordLength_8b; // 数据位长度为8位
USART_InitStruct.USART_StopBits = USART_StopBits_1; // 停止位为1位
USART_InitStruct.USART_Parity = USART_Parity_No; // 无奇偶校验
USART_InitStruct.USART_HardwareFlowControl = USART_HardwareFlowControl_None; // 无硬件流控制
USART_InitStruct.USART_Mode = USART_Mode_Rx | USART_Mode_Tx; // 同时使能接收和发送
USART_Init(USART1, &USART_InitStruct);
// 使能USART1
USART_Cmd(USART1, ENABLE);
}
void USART1_SendChar(char c) {
// 等待发送缓冲区为空
while (!(USART1->SR & USART_SR_TXE));
// 发送字符
USART1->DR = (c & 0xFF);
}
char USART1_GetChar(void) {
// 等待接收缓冲区非空
while (!(USART1->SR & USART_SR_RXNE));
// 返回接收到的字符
return (USART1->DR & 0xFF);
}
int main(void) {
char receivedChar;
// 初始化串口1
USART1_Init();
while (1) {
// 接收字符
receivedChar = USART1_GetChar();
// 发送接收到的字符
USART1_SendChar(receivedChar);
}
}
```
这段代码中,首先定义了 `USART1_Init()` 函数,用于初始化串口1的引脚和参数。然后定义了 `USART1_SendChar()` 和 `USART1_GetChar()` 函数,分别用于发送和接收单个字符。最后,在 `main()` 函数中,初始化串口1,并在一个无限循环中不断接收并发送字符。
请注意,这只是一个简单的示例代码,用于演示如何使用串口1进行数据收发。你可以根据自己的需求进行修改和扩展。
阅读全文
相关推荐

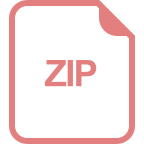
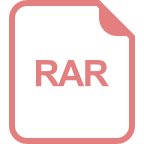
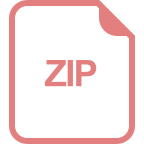
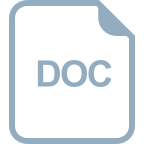








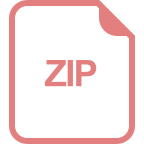