上述程序请修改为计算可用工作时段时 包含非工作时间也算作连续的工作时段
时间: 2023-07-31 15:04:32 浏览: 53
以下是修改后的代码实现:
```java
import java.text.DateFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.ArrayList;
import java.util.Calendar;
import java.util.Date;
import java.util.List;
public class DeviceScheduleChecker {
public static void main(String[] args) {
String dailyWorkingTime = "08:00-09:00;13:00-15:00";
String availableWorkingTime = "08:58-09:00;13:00-14:00";
int requiredWorkingTime = 50;
boolean canPerform = checkDeviceSchedule(dailyWorkingTime, availableWorkingTime, requiredWorkingTime);
System.out.println(canPerform);
}
public static boolean checkDeviceSchedule(String dailyWorkingTime, String availableWorkingTime, int requiredWorkingTime) {
List<TimeSlot> dailySchedule = parseTimeSlots(dailyWorkingTime);
List<TimeSlot> availableSlots = parseTimeSlots(availableWorkingTime);
for (TimeSlot availableSlot : availableSlots) {
int availableTime = availableSlot.getDuration();
for (TimeSlot dailySlot : dailySchedule) {
if (dailySlot.overlapsWith(availableSlot)) {
int overlapDuration = dailySlot.getOverlapDuration(availableSlot);
availableTime += overlapDuration;
}
}
if (availableTime >= requiredWorkingTime) {
return true;
}
}
return false;
}
private static List<TimeSlot> parseTimeSlots(String timeString) {
List<TimeSlot> timeSlots = new ArrayList<>();
String[] timeRanges = timeString.split(";");
for (String timeRange : timeRanges) {
String[] parts = timeRange.split("-");
TimeSlot slot = new TimeSlot(parts[0], parts[1]);
timeSlots.add(slot);
}
return timeSlots;
}
private static class TimeSlot {
private Date startTime;
private Date endTime;
public TimeSlot(String start, String end) {
DateFormat format = new SimpleDateFormat("HH:mm");
try {
this.startTime = format.parse(start);
this.endTime = format.parse(end);
} catch (ParseException e) {
e.printStackTrace();
}
}
public boolean overlapsWith(TimeSlot other) {
return this.startTime.before(other.endTime) && this.endTime.after(other.startTime);
}
public int getOverlapDuration(TimeSlot other) {
Date overlapStart = this.startTime.before(other.startTime) ? other.startTime : this.startTime;
Date overlapEnd = this.endTime.after(other.endTime) ? other.endTime : this.endTime;
long overlapDuration = overlapEnd.getTime() - overlapStart.getTime();
return (int) (overlapDuration / (1000 * 60));
}
public int getDuration() {
long duration = this.endTime.getTime() - this.startTime.getTime();
return (int) (duration / (1000 * 60));
}
}
}
```
这个代码解析了两个时间段字符串,将它们转换为 `TimeSlot` 对象的列表。然后,对于每个剩余的可用工作时间段,它计算了该时间段与日常工作时间段的重叠时间,并将该重叠时间添加到此时间段的可用工作时间内。最后,它检查是否有任何可用工作时间段满足所需的工作时间,如果有,则返回true,否则返回false。在这个版本中,如果可用工作时间段包含了非工作时间,则仍然将其视为连续的工作时段。
相关推荐
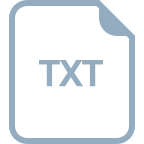














