词频统计并绘制词云图。
时间: 2023-08-20 21:05:02 浏览: 166
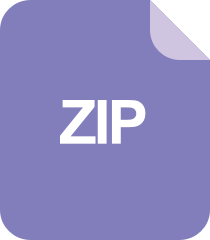
词频统计系统

好的,我可以使用Python中的nltk库和wordcloud库来实现词频统计和绘制词云图。以下是一个简单的代码示例:
```python
import nltk
from nltk.corpus import stopwords
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件
with open('example.txt', 'r') as f:
text = f.read()
# 分词
tokens = nltk.word_tokenize(text)
# 去除停用词
stop_words = set(stopwords.words('english'))
filtered_tokens = [word.lower() for word in tokens if word.lower() not in stop_words]
# 统计词频
freq_dist = nltk.FreqDist(filtered_tokens)
freq_dict = dict(freq_dist)
# 绘制词云图
wordcloud = WordCloud(width=800, height=800, background_color='white').generate_from_frequencies(freq_dict)
plt.figure(figsize=(8, 8), facecolor=None)
plt.imshow(wordcloud)
plt.axis('off')
plt.tight_layout(pad=0)
plt.show()
```
在这个例子中,我们首先读取一个文本文件并进行分词和去除停用词操作。然后,使用nltk库中的FreqDist类对单词进行计数,并将结果存储在一个字典中。最后,使用wordcloud库中的WordCloud类绘制词云图并显示出来。
请注意,这只是一个简单的示例,实际使用中可能需要进行更多的数据清洗和处理操作。
阅读全文
相关推荐
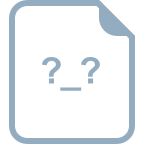















