python判断字典非空
时间: 2023-09-29 12:05:43 浏览: 90
要判断一个字典是否为空,可以使用以下方法:
1. 使用条件语句判断字典是否为空:
```python
my_dict = {}
if my_dict:
print("字典不为空")
else:
print("字典为空")
```
2. 使用`len()`函数判断字典的长度是否为0:
```python
my_dict = {}
if len(my_dict) != 0:
print("字典不为空")
else:
print("字典为空")
```
这两种方法都可以判断字典是否为空,根据实际情况选择适合的方法即可。
相关问题
python 非空判断
在 Python 中,可以通过以下方法进行非空判断:
1. 使用 if 语句判断变量是否为空,例如:
```
my_var = "Hello World"
if my_var:
print("my_var is not empty")
```
2. 使用 len() 函数判断容器对象(如字符串、列表、元组、字典等)是否为空,例如:
```
my_list = [1, 2, 3]
if len(my_list) > 0:
print("my_list is not empty")
```
3. 判断字符串、列表、元组、字典等是否为空,例如:
```
my_str = ""
if not my_str:
print("my_str is empty")
my_dict = {}
if not my_dict:
print("my_dict is empty")
```
注意,在 Python 中,以下值被认为是 False:False、None、0、空字符串、空列表、空元组、空字典。其余值被认为是 True。因此,可以使用 not 运算符来判断一个值是否为 False。
python中字典的定义
在Python中,字典是一种无序的数据结构,用于存储键值对。字典的定义可以通过以下几种方式实现:
1. 使用花括号{}创建空字典:`d = {}` 或 `d = dict()`
2. 创建非空的字典:`dic = {'key1': 'value1', 'key2': 'value2', ...}`
3. 使用`dict()`函数和元组创建字典:`dic = dict(('key1', 'value1'), ('key2', 'value2'), ...)`
需要注意的是,字典的键是唯一的,如果重复,最后的一个键值对会替换前面的。此外,可以使用`in`或`not in`运算符来判断字典是否包含指定的键。对于字典的常用方法,可以使用`clear()`方法清空字典中的所有键值对,使用`get()`方法根据键获取对应的值,使用`update()`方法更新字典中的键值对,使用`items()`、`keys()`和`values()`方法分别获取字典中的所有键值对、所有键和所有值。另外,可以使用`pop()`方法获取指定键对应的值,并删除该键值对。\[1\]\[2\]\[3\]
#### 引用[.reference_title]
- *1* [【python入门篇——11】字典(dict)详解](https://blog.csdn.net/weixin_45014379/article/details/125641819)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* [python字典](https://blog.csdn.net/qq_41545638/article/details/107304803)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
- *3* [python--字典](https://blog.csdn.net/weixin_45326288/article/details/117371482)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^control,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
相关推荐
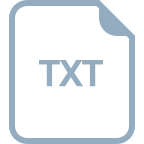












