利用vue2和elementui 做一个table表格有增删改查 状态开关 展开
时间: 2024-04-12 12:27:31 浏览: 176
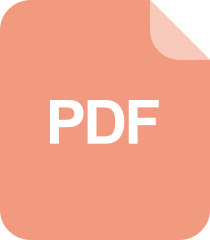
vue.js+Element实现表格里的增删改查
你可以按照以下步骤使用Vue 2和Element UI创建一个具有增删改查、状态开关和展开功能的表格:
1. 首先,确保你已经安装了Vue和Element UI库。你可以使用npm或yarn来安装它们:
```bash
npm install vue@2.6.14
npm install element-ui@2.15.6
```
2. 创建一个Vue组件,用于显示表格和处理相关逻辑。在该组件中,你可以定义数据、方法和计算属性。
```vue
<template>
<div>
<el-table :data="tableData" style="width: 100%">
<el-table-column label="编号" prop="id"></el-table-column>
<el-table-column label="姓名" prop="name"></el-table-column>
<el-table-column label="状态">
<template slot-scope="scope">
<el-switch v-model="scope.row.status"></el-switch>
</template>
</el-table-column>
<el-table-column>
<template slot-scope="scope">
<el-button @click="handleExpand(scope.row)" type="text">展开</el-button>
</template>
</el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button @click="handleEdit(scope.row)" type="text">编辑</el-button>
<el-button @click="handleDelete(scope.row)" type="text">删除</el-button>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [
{ id: 1, name: '张三', status: false },
{ id: 2, name: '李四', status: true },
{ id: 3, name: '王五', status: true }
]
};
},
methods: {
handleEdit(row) {
// 处理编辑操作
},
handleDelete(row) {
// 处理删除操作
},
handleExpand(row) {
// 处理展开操作
}
}
};
</script>
```
3. 在你的Vue入口文件中,引入Vue和Element UI,并注册Element UI组件。
```javascript
import Vue from 'vue';
import ElementUI from 'element-ui';
import 'element-ui/lib/theme-chalk/index.css';
import App from './App.vue';
Vue.use(ElementUI);
new Vue({
render: h => h(App)
}).$mount('#app');
```
这样,你就可以在Vue应用中使用Element UI的表格组件,并实现增删改查、状态开关和展开功能了。你可以根据需要在`handleEdit`、`handleDelete`和`handleExpand`方法中编写相应的逻辑。
阅读全文
相关推荐
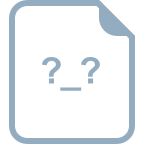
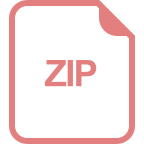
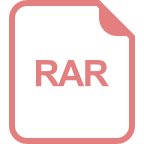
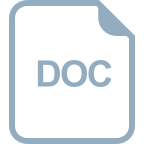
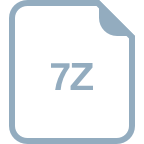
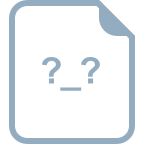
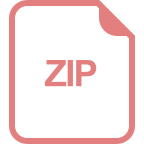
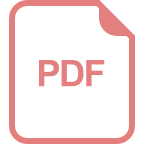
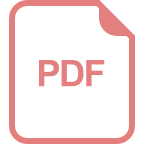
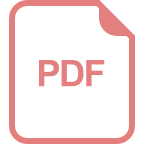
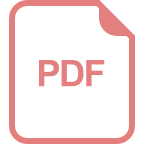
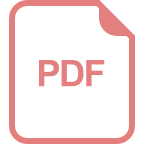
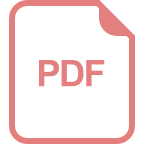
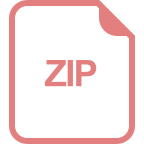
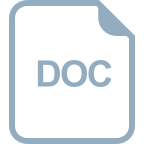
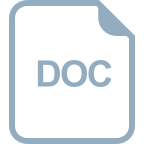
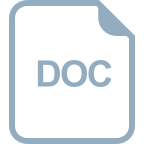