Write a function pair_numbers that takes in two lists of numbers with the same length and returns a list of tuples. Example: pair_numbers([1],[1]) = [(1,1)] pair_numbers([1,2],[3,4]) = [(1,3),(1,4),(2,3),(2,4)] pair_numbers([],[]) = []
时间: 2023-12-28 16:04:51 浏览: 118
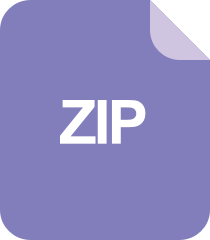
boostdesc-bgm.i文件,使用opencv编译时需要下载的文件
Certainly! Here is the implementation of the `pair_numbers` function:
```python
def pair_numbers(list1, list2):
pairs = []
for num1 in list1:
for num2 in list2:
pairs.append((num1, num2))
return pairs
```
The `pair_numbers` function takes in two lists of numbers, `list1` and `list2`, with the same length. It creates an empty list called `pairs` to store the resulting tuples. It then iterates over each element in `list1` and `list2` using nested loops. For each combination of elements, it creates a tuple `(num1, num2)` and appends it to the `pairs` list. Finally, it returns the `pairs` list containing all the tuples.
Here are a few examples of using the `pair_numbers` function:
```python
print(pair_numbers([1], [1])) # Output: [(1, 1)]
print(pair_numbers([1, 2], [3, 4])) # Output: [(1, 3), (1, 4), (2, 3), (2, 4)]
print(pair_numbers([], [])) # Output: []
```
In the first example, it pairs the single elements `[1]` and `[1]`, resulting in `[(1, 1)]`. In the second example, it pairs each element from the first list with each element from the second list, resulting in `[(1, 3), (1, 4), (2, 3), (2, 4)]`. In the third example, as both lists are empty, it returns an empty list `[]`.
阅读全文
相关推荐
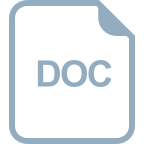
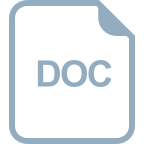
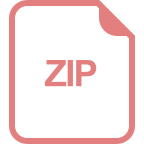
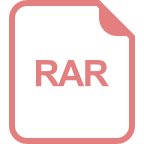
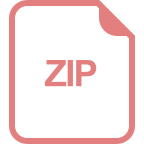
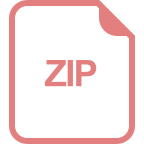
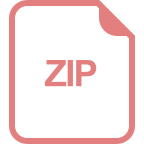
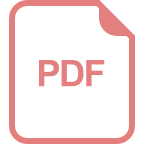
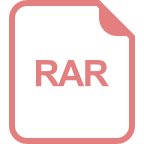
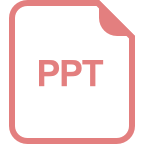
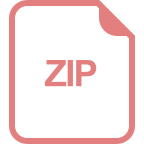
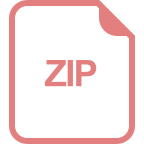
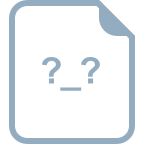
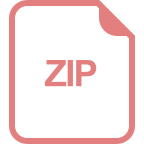