XGBoost的python实现代码并逐步解释
时间: 2024-06-11 08:02:42 浏览: 202
XGBoost是一种高效、灵活并且可移植的梯度提升框架。它在很多数据科学竞赛中都取得了不错的成绩,也被广泛应用于各种实际问题中。在Python中,可以使用XGBoost的Python包来使用该框架。
以下是使用Python实现XGBoost的基本步骤:
1. 导入必要的库和数据:首先需要导入所需的Python库,如numpy和pandas等,以及需要进行模型训练和预测的数据。
```
import numpy as np
import pandas as pd
import xgboost as xgb
# 导入数据
data = pd.read_csv('data.csv')
```
2. 数据预处理:对于许多数据集,需要进行一些数据清洗和特征工程处理来提高模型性能。例如,可能需要对缺失值进行填充、进行特征选择、进行特征缩放等。
```
# 填充缺失值
data.fillna(value=-999, inplace=True)
# 特征选择和特征缩放
X = data.drop('target', axis=1)
y = data['target']
# 特征缩放
from sklearn.preprocessing import StandardScaler
scaler = StandardScaler()
X = scaler.fit_transform(X)
```
3. 划分训练集和测试集:将数据划分为训练集和测试集,以便评估模型性能。
```
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
```
4. 训练模型:使用XGBoost训练模型,并根据需要设置各种参数。
```
# 设置参数
params = {
'objective': 'binary:logistic',
'max_depth': 3,
'learning_rate': 0.1,
'silent': True,
'n_estimators': 100,
'gamma': 0,
'min_child_weight': 1,
'subsample': 1,
'colsample_bytree': 1,
'scale_pos_weight': 1,
'seed': 0,
'nthread': -1,
}
# 训练模型
dtrain = xgb.DMatrix(X_train, label=y_train)
dtest = xgb.DMatrix(X_test, label=y_test)
model = xgb.train(params, dtrain, num_boost_round=10)
```
5. 模型预测:使用训练好的模型对测试集进行预测,并评估模型性能。
```
# 预测并评估性能
y_pred = model.predict(dtest)
threshold = 0.5
y_pred[y_pred > threshold] = 1
y_pred[y_pred <= threshold] = 0
from sklearn.metrics import accuracy_score, confusion_matrix, classification_report
print('Accuracy:', accuracy_score(y_test, y_pred))
print('Confusion Matrix:', confusion_matrix(y_test, y_pred))
print('Classification Report:', classification_report(y_test, y_pred))
```
以上就是使用Python实现XGBoost的基本步骤。通过调整参数和进行特征工程等方法,可以进一步提高模型性能。
阅读全文
相关推荐
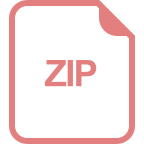
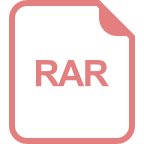
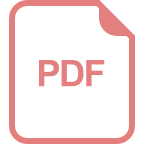










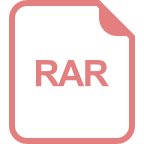
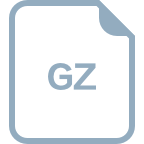
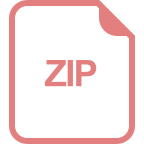
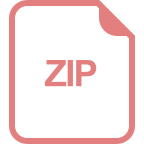
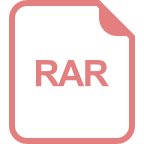
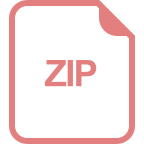