用Python做一个模拟购物过程,包括商品选择、价格计算
时间: 2024-09-09 10:14:49 浏览: 75
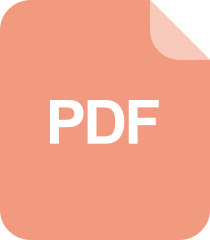
基于Python的购物中心人流分布计算机模拟——以厦门湖里万达广场为例.pdf

在Python中,我们可以创建一个简单的模拟购物车系统来演示这个过程。首先,我们定义一个商品类(Product),包含基本信息如名称(name)、单价(price)和库存(stock)。然后,我们创建一个购物车类(ShoppingCart),其中可以添加商品、删除商品以及计算总价。
```python
class Product:
def __init__(self, name, price, stock):
self.name = name
self.price = price
self.stock = stock
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, product, quantity=1):
if product.stock > 0:
product.stock -= quantity
self.items.append((product, quantity))
else:
print(f"商品 '{product.name}' 没有库存了")
def remove_item(self, product_name, quantity=1):
for item in self.items:
if item[0].name == product_name:
if quantity <= item[1]:
item[1] -= quantity
product.stock += quantity
if item[1] == 0:
self.items.remove(item)
else:
product.stock -= item[1]
self.items.remove(item)
break
else:
print(f"找不到商品 '{product_name}'")
def calculate_total(self):
total_price = sum([item[0].price * item[1] for item in self.items])
return total_price
# 示例用法
apple = Product("苹果", 5, 10)
cart = ShoppingCart()
cart.add_item(apple, 3) # 添加3个苹果
print(f"购物车总价:{cart.calculate_total()} 元")
# 删除一个苹果
cart.remove_item("苹果", 2)
print(f"购物车剩余商品及总价:{cart.items},{cart.calculate_total()} 元")
阅读全文
相关推荐
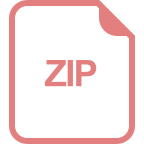
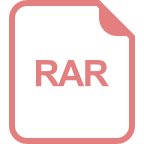
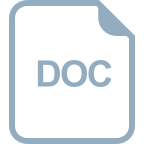


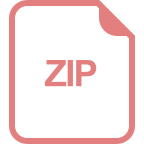











