使用COCOS TS编写一个2D游戏的弹簧代码
时间: 2024-09-11 19:11:17 浏览: 41
在使用Cocos Creator(COCOS TS通常指的是Cocos Creator中的TypeScript环境)编写一个2D游戏时,创建一个弹簧效果可以通过物理引擎(如Cocos内置的Box2D)或者通过编程方式实现。这里提供一个简单的弹簧效果实现思路:
1. 创建一个弹簧类(Spring),该类负责管理弹簧的行为和属性。
2. 在弹簧类中定义弹簧的锚点,即弹簧固定的位置。
3. 使用游戏循环更新弹簧的长度,通过控制弹簧两端对象的位置来模拟弹簧的伸缩效果。
4. 可以设置弹簧的自然长度、弹性系数和阻尼系数等参数,来模拟不同的物理特性。
示例代码(TypeScript):
```typescript
class Spring {
constructor(anchor: cc.Node, target: cc.Node, naturalLength: number, elasticity: number, damping: number) {
this.anchor = anchor;
this.target = target;
this.naturalLength = naturalLength;
this.elasticity = elasticity;
this.damping = damping;
this.length = this.target.position.distance(this.anchor.position);
}
update() {
let direction = cc.v2(this.target.position).sub(this.anchor.position).normalize();
let deltaLength = this.length - this.naturalLength;
let force = deltaLength * this.elasticity;
force = direction.mul(force);
force = force.add(this.target.velocity.mul(-this.damping));
this.target.runAction(cc.moveTo(0.1, this.anchor.position.x + direction.x * this.naturalLength, this.anchor.position.y + direction.y * this.naturalLength).easing(cc.Easing.QUADRATIC_IN_OUT));
this.target.velocity = this.target.velocity.add(force);
this.length = this.target.position.distance(this.anchor.position);
}
}
// 使用示例:
let spring = new Spring(anchorNode, targetNode, 50, 0.5, 0.7);
this.updateSpring = () => {
spring.update();
}
Director.getInstance().schedule(this.updateSpring);
```
在这个例子中,我们创建了一个Spring类,它负责根据弹簧两端节点(anchor和target)的位置来模拟弹簧的物理行为。`update`方法会在每一帧调用,更新弹簧的目标节点的位置。这里的代码是一个非常简化的示例,实际游戏中可能需要更复杂的物理模拟和动画效果。
阅读全文
相关推荐
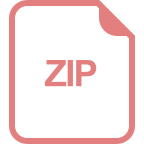
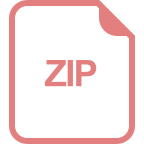
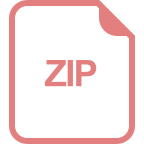
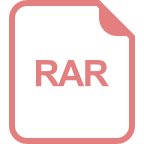
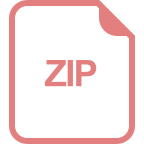
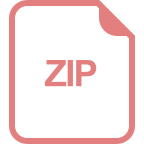
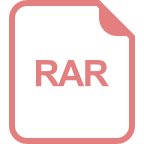
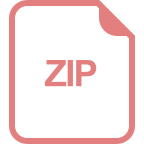
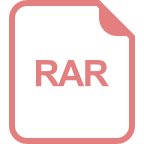
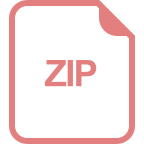
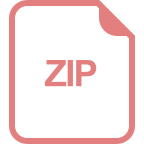
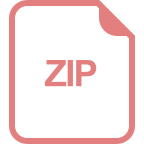
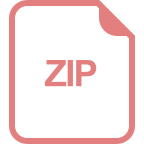
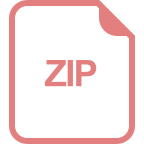
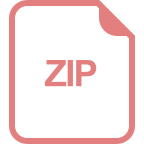
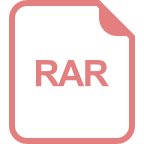