用c++编写一个酒店客房管理系统
时间: 2023-06-25 16:04:56 浏览: 49
这里给出一个简单的酒店客房管理系统的实现,包括客房信息的录入、查询、修改和删除功能。
```c++
#include <iostream>
#include <vector>
#include <fstream>
#include <string>
using namespace std;
struct Room {
int id; // 客房号码
string type; // 房型
int price; // 价格
bool status; // 房间状态:true-已预订,false-未预订
};
vector<Room> rooms; // 客房信息存储
// 读取客房信息
void readRooms() {
ifstream fin("rooms.txt");
if (!fin) {
cout << "文件打开失败!" << endl;
return;
}
while (!fin.eof()) {
Room room;
fin >> room.id >> room.type >> room.price >> room.status;
if (fin.fail()) {
break;
}
rooms.push_back(room);
}
fin.close();
}
// 显示所有客房信息
void showRooms() {
cout << "客房信息如下:" << endl;
for (int i = 0; i < rooms.size(); i++) {
cout << rooms[i].id << "\t" << rooms[i].type << "\t" << rooms[i].price << "\t";
if (rooms[i].status) {
cout << "已预订" << endl;
} else {
cout << "未预订" << endl;
}
}
}
// 添加客房信息
void addRoom() {
Room room;
cout << "请输入客房号码:";
cin >> room.id;
cout << "请输入房型:";
cin >> room.type;
cout << "请输入价格:";
cin >> room.price;
room.status = false;
rooms.push_back(room);
cout << "客房信息添加成功!" << endl;
}
// 查询客房信息
void searchRoom() {
int id;
cout << "请输入要查询的客房号码:";
cin >> id;
for (int i = 0; i < rooms.size(); i++) {
if (rooms[i].id == id) {
cout << rooms[i].id << "\t" << rooms[i].type << "\t" << rooms[i].price << "\t";
if (rooms[i].status) {
cout << "已预订" << endl;
} else {
cout << "未预订" << endl;
}
return;
}
}
cout << "未找到指定客房!" << endl;
}
// 修改客房信息
void modifyRoom() {
int id;
cout << "请输入要修改的客房号码:";
cin >> id;
for (int i = 0; i < rooms.size(); i++) {
if (rooms[i].id == id) {
cout << "请输入新的房型:";
cin >> rooms[i].type;
cout << "请输入新的价格:";
cin >> rooms[i].price;
cout << "客房信息修改成功!" << endl;
return;
}
}
cout << "未找到指定客房!" << endl;
}
// 删除客房信息
void deleteRoom() {
int id;
cout << "请输入要删除的客房号码:";
cin >> id;
for (int i = 0; i < rooms.size(); i++) {
if (rooms[i].id == id) {
rooms.erase(rooms.begin() + i);
cout << "客房信息删除成功!" << endl;
return;
}
}
cout << "未找到指定客房!" << endl;
}
// 保存客房信息
void saveRooms() {
ofstream fout("rooms.txt");
if (!fout) {
cout << "文件打开失败!" << endl;
return;
}
for (int i = 0; i < rooms.size(); i++) {
fout << rooms[i].id << "\t" << rooms[i].type << "\t" << rooms[i].price << "\t" << rooms[i].status << endl;
}
fout.close();
}
int main() {
readRooms();
int choice;
while (true) {
cout << "请选择要进行的操作:" << endl;
cout << "1. 显示所有客房信息" << endl;
cout << "2. 添加客房信息" << endl;
cout << "3. 查询客房信息" << endl;
cout << "4. 修改客房信息" << endl;
cout << "5. 删除客房信息" << endl;
cout << "6. 退出系统" << endl;
cin >> choice;
switch (choice) {
case 1:
showRooms();
break;
case 2:
addRoom();
break;
case 3:
searchRoom();
break;
case 4:
modifyRoom();
break;
case 5:
deleteRoom();
break;
case 6:
saveRooms();
return 0;
default:
cout << "输入有误,请重新输入!" << endl;
break;
}
}
return 0;
}
```
客房信息保存在文本文件 "rooms.txt" 中,每行包含客房号码、房型、价格和状态。客房信息的读取和保存使用了文件输入输出流。客房信息存储使用了 vector 容器。客房状态采用了布尔类型,true 表示已预订,false 表示未预订。客房管理系统提供了添加、查询、修改和删除客房信息的功能。
相关推荐
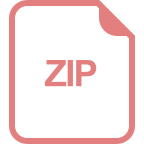
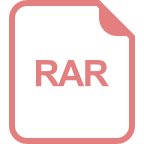
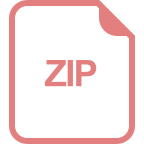














