蚁群 二维路径规划 python
时间: 2024-01-14 16:00:50 浏览: 201
蚁群算法是一种启发式算法,通过模拟蚂蚁在寻找食物过程中的行为,来寻找最优解。在二维路径规划中,蚁群算法可以被用来找到最短路径,例如在地图上寻找最短路径或者在电路板上寻找最佳布线。Python是一种高级编程语言,它的简洁性和可读性使得它成为了很多数据科学和算法实现的首选。
在使用Python实现蚁群算法进行二维路径规划时,首先需要定义蚂蚁的行为规则,例如蚂蚁如何在路径上移动,并且在移动过程中如何更新信息素。然后需要设计一个二维的路径规划问题,并使用Python来表示这个问题的地图和各个节点之间的距离。接下来,可以使用Python编写蚁群算法的代码,利用已有的库和工具来实现蚂蚁寻找最短路径的过程。最后,通过不断迭代蚂蚁的移动和路径信息素的更新,直到达到停止条件为止,就可以得到最优的路径规划结果。
Python具有丰富的库和工具,例如numpy、matplotlib和scipy等,可以辅助实现蚁群算法的二维路径规划。在编写代码时,可以充分利用这些库来简化算法实现的过程,并且通过可视化工具来直观地展示最优路径的搜索过程和结果。因此,利用Python实现蚁群算法进行二维路径规划不仅可以高效地解决问题,而且还可以得到直观的结果展示。
相关问题
蚁群算法python路径规划
蚁群算法(Ant Colony Optimization,简称ACO)是一种基于蚂蚁群体智能的启发式搜索算法,常用于求解路径规划问题。下面给出一个简单的Python实现,用于解决一个简单的路径规划问题。
首先,我们需要定义一个问题:假设有一个二维的迷宫,其中包含起点和终点以及一些障碍物,我们需要找到从起点到终点的最短路径,同时要避开障碍物。我们可以将迷宫表示为一个二维数组,其中0表示可通行的空间,1表示障碍物。
```python
import numpy as np
# 定义迷宫,0表示可通行的空间,1表示障碍物
maze = np.array([
[0, 0, 0, 0, 0, 0, 0, 1],
[0, 1, 1, 1, 0, 1, 0, 1],
[0, 0, 0, 0, 0, 0, 0, 1],
[0, 1, 0, 1, 1, 1, 0, 0],
[0, 1, 0, 0, 0, 0, 1, 0],
[0, 1, 1, 1, 0, 1, 0, 0],
[0, 0, 0, 0, 0, 0, 0, 1],
[0, 1, 1, 1, 0, 1, 0, 0]
])
# 定义起点和终点
start = (0, 0)
end = (7, 7)
```
接下来,我们需要定义一个蚂蚁类,用于实现蚂蚁的移动和路径更新:
```python
class Ant:
def __init__(self, start, end, maze):
self.position = start
self.path = [start]
self.end = end
self.maze = maze
def move(self, alpha=1, beta=2, Q=100, pheromone=0.1):
while self.position != self.end:
row, col = self.position
neighbors = []
# 获取周围可通行的位置
for i in range(row-1, row+2):
for j in range(col-1, col+2):
if i >= 0 and j >= 0 and i < len(maze) and j < len(maze[0]) and maze[i][j] == 0 and (i, j) not in self.path:
neighbors.append((i, j))
# 计算每个邻居的概率
probabilities = []
for neighbor in neighbors:
ph = pheromone ** alpha
distance = ((neighbor[0] - self.end[0]) ** 2 + (neighbor[1] - self.end[1]) ** 2) ** 0.5
heuristic = distance ** beta
probabilities.append(ph * heuristic)
probabilities = np.array(probabilities)
probabilities /= probabilities.sum()
# 根据概率选择下一个位置
next_position = neighbors[np.random.choice(len(neighbors), p=probabilities)]
self.path.append(next_position)
self.position = next_position
# 更新路径上的信息素
for i in range(len(self.path)-1):
row1, col1 = self.path[i]
row2, col2 = self.path[i+1]
maze[row1][col1] += Q / ((row2-row1)**2 + (col2-col1)**2)
```
在蚂蚁类中,我们首先定义了蚂蚁的起点、终点、当前位置和已经走过的路径。然后,我们定义了一个move方法,用于实现蚂蚁的移动和路径更新。在move方法中,我们首先获取当前位置周围可通行的位置,然后计算每个邻居的概率,根据概率选择下一个位置。在选择下一个位置后,我们更新蚂蚁的位置和已经走过的路径。最后,我们根据路径上的信息素,更新迷宫中的信息素。
接下来,我们可以使用多个蚂蚁来搜索最短路径:
```python
# 初始化信息素
pheromone = np.ones_like(maze) * 0.1
# 定义参数
alpha = 1
beta = 2
Q = 100
n_ants = 100
n_iterations = 100
# 开始搜索
for iteration in range(n_iterations):
ants = [Ant(start, end, maze) for _ in range(n_ants)]
for ant in ants:
ant.move(alpha, beta, Q, pheromone)
# 更新信息素
pheromone *= 0.9
for ant in ants:
for i in range(len(ant.path)-1):
row1, col1 = ant.path[i]
row2, col2 = ant.path[i+1]
pheromone[row1][col1] += Q / ((row2-row1)**2 + (col2-col1)**2)
# 更新最佳路径
best_path = None
best_distance = float('inf')
for ant in ants:
distance = sum(((ant.path[i][0]-ant.path[i+1][0])**2 + (ant.path[i][1]-ant.path[i+1][1])**2)**0.5 for i in range(len(ant.path)-1))
if distance < best_distance:
best_distance = distance
best_path = ant.path
# 输出最佳路径
print(best_path)
```
在搜索过程中,我们首先初始化信息素,并定义了一些参数,包括alpha、beta、Q、蚂蚁数量和迭代次数。然后,我们开始迭代搜索,每次迭代都用多个蚂蚁来搜索路径,并更新信息素。在每次迭代结束后,我们更新最佳路径。最后输出最佳路径即可。
完整代码如下:
给出一个蚁群算法的旅行商Python
以下是一个蚁群算法的旅行商Python代码示例:
```
import random
import math
class AntColony:
def __init__(self, distance_matrix, num_ants, num_iterations, alpha, beta, evaporation_rate, q):
self.distance_matrix = distance_matrix
self.num_ants = num_ants
self.num_iterations = num_iterations
self.alpha = alpha
self.beta = beta
self.evaporation_rate = evaporation_rate
self.q = q
self.num_cities = len(distance_matrix)
self.pheromone_matrix = [[1 / (self.num_cities * self.num_cities) for j in range(self.num_cities)] for i in range(self.num_cities)]
def run(self):
best_path = []
best_distance = math.inf
for i in range(self.num_iterations):
paths = self.generate_paths()
distances = [self.get_distance(path) for path in paths]
for ant, path in enumerate(paths):
if distances[ant] < best_distance:
best_distance = distances[ant]
best_path = path
self.update_pheromone(path, distances[ant])
self.evaporate_pheromone()
return best_path, best_distance
def generate_paths(self):
paths = []
for ant in range(self.num_ants):
path = [random.randint(0, self.num_cities - 1)]
while len(path) < self.num_cities:
next_city = self.choose_next_city(path)
path.append(next_city)
paths.append(path)
return paths
def choose_next_city(self, path):
current_city = path[-1]
unvisited_cities = set(range(self.num_cities)) - set(path)
if not unvisited_cities:
return path[0]
probabilities = []
for city in unvisited_cities:
pheromone = self.pheromone_matrix[current_city][city]
distance = self.distance_matrix[current_city][city]
probability = math.pow(pheromone, self.alpha) * math.pow(1 / distance, self.beta)
probabilities.append((city, probability))
total_probability = sum(probability for _, probability in probabilities)
normalized_probabilities = [(city, probability / total_probability) for city, probability in probabilities]
next_city = random.choices([city for city, _ in normalized_probabilities], [probability for _, probability in normalized_probabilities])[0]
return next_city
def get_distance(self, path):
distance = 0
for i in range(self.num_cities - 1):
distance += self.distance_matrix[path[i]][path[i + 1]]
distance += self.distance_matrix[path[-1]][path[0]]
return distance
def update_pheromone(self, path, distance):
pheromone_deposit = self.q / distance
for i in range(self.num_cities - 1):
current_city, next_city = path[i], path[i + 1]
self.pheromone_matrix[current_city][next_city] += pheromone_deposit
self.pheromone_matrix[next_city][current_city] += pheromone_deposit
start_city, end_city = path[-1], path[0]
self.pheromone_matrix[start_city][end_city] += pheromone_deposit
self.pheromone_matrix[end_city][start_city] += pheromone_deposit
def evaporate_pheromone(self):
for i in range(self.num_cities):
for j in range(self.num_cities):
self.pheromone_matrix[i][j] *= (1 - self.evaporation_rate)
```
这个类可以用以下方式来运行:
```
distance_matrix = [[0, 2, 9, 10],
[1, 0, 6, 4],
[15, 7, 0, 8],
[6, 3, 12, 0]]
colony = AntColony(distance_matrix, num_ants=10, num_iterations=100, alpha=1, beta=2, evaporation_rate=0.5, q=100)
best_path, best_distance = colony.run()
print('Best path:', best_path)
print('Best distance:', best_distance)
```
这里的`distance_matrix`是一个二维列表,包含每个城市之间的距离。这里的示例包含了4个城市,但这个算法可以适用于任意数量的城市。其他参数中,`num_ants`表示蚂蚁的数量,`num_iterations`表示迭代次数,`alpha`和`beta`表示控制信息素和距离之间权重的参数,`evaporation_rate`表示信息素蒸发率,`q`表示信息素沉积强度。最后,`run()`函数返回最佳路径和最佳距离。
阅读全文
相关推荐
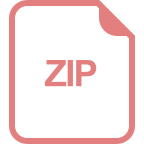
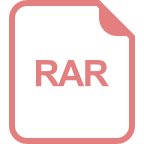
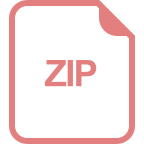
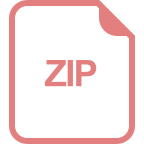
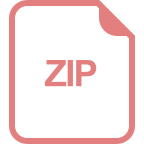
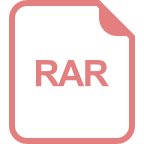
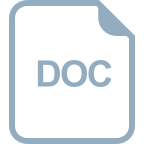
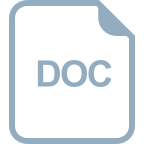
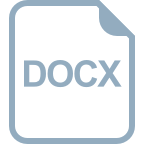
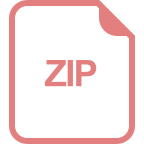
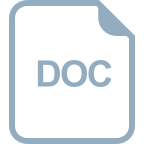
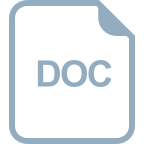
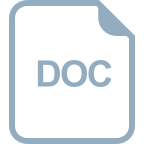
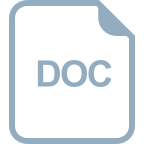
