使用intellij和spring boot,用restful api设计并实现shipping and transportation services,这些api可以直接用postapi测试,结合spring mvc,jpa,mysql和jwt
时间: 2023-12-10 11:37:28 浏览: 24
好的,让我为您提供一些指导。
首先,您需要安装IntelliJ IDEA并设置Spring Boot开发环境。接下来,您可以使用Spring Initializr创建一个新的Spring Boot项目,其中包括Spring MVC和Spring Data JPA依赖项。
一旦您的项目设置完成,您可以开始定义RESTful API。您可以使用@RestController注释定义控制器类,并使用@RequestMapping注释定义处理不同HTTP请求的方法。例如:
```
@RestController
@RequestMapping("/api")
public class ShippingController {
@Autowired
private ShippingService shippingService;
@GetMapping("/shipments")
public List<Shipment> getAllShipments() {
return shippingService.getAllShipments();
}
@PostMapping("/shipments")
public Shipment createShipment(@RequestBody Shipment shipment) {
return shippingService.createShipment(shipment);
}
// other methods
}
```
在这个例子中,我们定义了一个ShippingController类,它处理与运输相关的HTTP请求。我们使用@Autowired注释将ShippingService注入到控制器中,以便我们可以使用该服务来处理业务逻辑。我们还定义了一个@GetMapping注释的方法,该方法返回所有运输记录,并定义了一个@PostMapping注释的方法,该方法创建新的运输记录。
接下来,您需要实现ShippingService类,该类将处理所有业务逻辑。您可以使用Spring Data JPA来处理与数据库的交互。例如:
```
@Service
public class ShippingService {
@Autowired
private ShipmentRepository shipmentRepository;
public List<Shipment> getAllShipments() {
return shipmentRepository.findAll();
}
public Shipment createShipment(Shipment shipment) {
return shipmentRepository.save(shipment);
}
// other methods
}
```
在这个例子中,我们定义了一个ShippingService类,该类使用@Autowired注释将ShipmentRepository注入到服务中。我们还定义了一个getAllShipments方法,该方法使用findAll方法从数据库中检索所有运输记录,并定义了一个createShipment方法,该方法使用save方法将新的运输记录保存到数据库中。
最后,您可以使用Postman等工具测试您的API。您还可以为您的API实现JWT身份验证,以确保只有经过身份验证的用户才能访问受保护的资源。
相关推荐
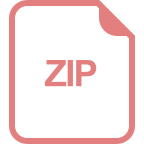
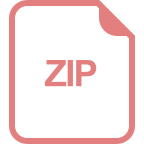
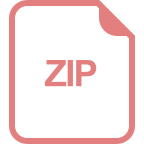














