https://www.lihuamuye.cn/tw/v1/public/sample/exportSample 这是一个下载excel文件的接口,请用vue+axios调用该接口并在前端将接口下载的excel文件重命名为abc.excel,给出相应的代码
时间: 2023-09-16 15:05:59 浏览: 192
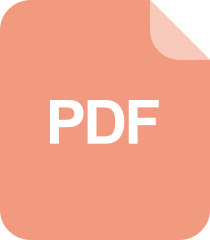
vue使用axios实现excel文件下载的功能
### 回答1:
可以使用下面的代码实现:axios({
method: 'get',
url: 'https://www.lihuamuye.cn/tw/v1/public/sample/exportSample',
responseType: 'blob' // 表明返回服务器返回的数据类型
}).then(res => {
const content = res.data
const blob = new Blob([content])
const fileName = 'abc.xlsx'
if ('download' in document.createElement('a')) {
// 非IE下载
const elink = document.createElement('a')
elink.download = fileName
elink.style.display = 'none'
elink.href = URL.createObjectURL(blob)
document.body.appendChild(elink)
elink.click()
URL.revokeObjectURL(elink.href) // 释放URL 对象
document.body.removeChild(elink)
} else {
// IE10+下载
navigator.msSaveBlob(blob, fileName)
}
})
### 回答2:
在Vue中使用axios调用接口并实现前端下载excel文件并重命名为abc.excel的代码如下:
1. 首先,安装axios依赖:
```bash
npm install axios --save
```
2. 在Vue组件中引入axios库:
```javascript
import axios from 'axios';
```
3. 在需要调用接口的方法中,使用axios发送GET请求并处理返回的文件流:
```javascript
export default {
methods: {
downloadExcelFile() {
const url = 'https://www.lihuamuye.cn/tw/v1/public/sample/exportSample'; // 接口地址
const filename = 'abc.excel'; // 下载的文件名
axios.get(url, {
responseType: 'blob' // 设置响应类型为blob(二进制文件)
})
.then(response => {
const blob = new Blob([response.data]); // 创建一个blob对象,用于存储返回的文件流
if (typeof window.navigator.msSaveBlob !== 'undefined') {
// 兼容IE浏览器
window.navigator.msSaveBlob(blob, filename);
} else {
// 创建一个下载链接
const link = document.createElement('a');
const url = URL.createObjectURL(blob);
link.href = url;
link.download = filename;
// 模拟点击下载链接
link.click();
// 释放创建的URL对象
URL.revokeObjectURL(url);
}
})
.catch(error => {
console.log(error);
});
}
}
}
```
4. 在模板中调用下载方法:
```html
<template>
<div>
<button @click="downloadExcelFile">下载Excel</button>
</div>
</template>
```
这样,当用户点击按钮时,就会调用接口并下载命名为abc.excel的文件。
### 回答3:
在Vue项目中使用axios调用接口并下载excel文件并重命名的代码如下:
```javascript
import axios from 'axios';
// 定义一个方法来下载excel文件
function downloadExcel() {
axios({
url: 'https://www.lihuamuye.cn/tw/v1/public/sample/exportSample',
method: 'GET',
responseType: 'blob', // 设置responseType为blob,以支持下载文件
})
.then((response) => {
const fileURL = window.URL.createObjectURL(new Blob([response.data])); // 创建一个文件URL对象
const link = document.createElement('a'); // 创建一个a标签
link.href = fileURL; // 设置a标签的href属性为文件URL
link.setAttribute('download', 'abc.xlsx'); // 设置文件下载的默认文件名为abc.xlsx
document.body.appendChild(link); // 将a标签添加到页面的body中
link.click(); // 模拟点击a标签进行下载
document.body.removeChild(link); // 下载完成后移除这个a标签
})
.catch((error) => {
console.log(error);
});
}
export default {
name: 'App',
methods: {
handleDownload() {
downloadExcel(); // 调用下载excel文件的方法
},
},
};
```
在Vue组件中,可以将该代码放在一个方法中,例如`handleDownload`,然后在相应的按钮或事件中调用这个方法,例如`<button @click="handleDownload">下载</button>`。当用户点击这个按钮时,将会触发调用接口下载excel文件,并在前端将其重命名为"abc.excel"。
阅读全文
相关推荐
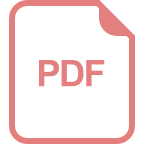
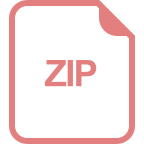
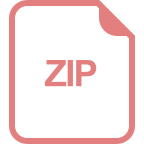
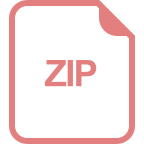
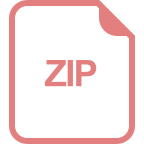
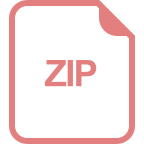
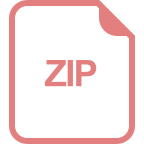
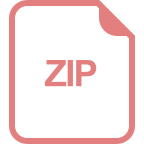
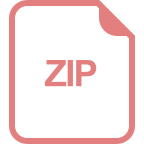
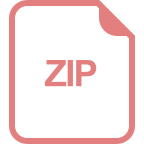
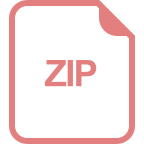
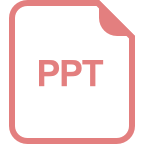
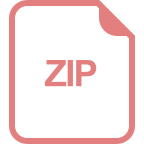
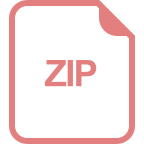
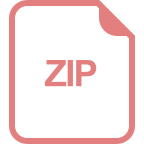
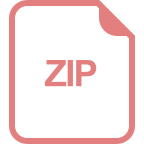