# -*-coding:utf-8 -*- import numpy as np from scipy import interpolate import pylab as pl x = np.linspace(0, 10, 11) # x=[ 0. 1. 2. 3. 4. 5. 6. 7. 8. 9. 10.] y = np.sin(x) xnew = np.linspace(0, 10, 101) pl.plot(x, y, "ro") for kind in ["nearest", "zero", "slinear", "quadratic", "cubic"]: # 插值方式 # "nearest","zero"为阶梯插值 # slinear 线性插值 # "quadratic","cubic" 为2阶、3阶B样条曲线插值 f = interpolate.interp1d(x, y, kind=kind) # ‘slinear’, ‘quadratic’ and ‘cubic’ refer to a spline interpolation of first, second or third order) ynew = f(xnew) pl.plot(xnew, ynew, label=str(kind)) pl.legend(loc="lower right") pl.show()请将这段代码改成在2006年到2021年间人口population = [113,111,113,113,117,121,125,130,136,143,140,127,120,92,79]
时间: 2023-11-28 08:05:02 浏览: 221
代码改动如下:
```python
import numpy as np
from scipy import interpolate
import matplotlib.pyplot as plt
year = np.linspace(2006, 2021, 15)
population = [113, 111, 113, 113, 117, 121, 125, 130, 136, 143, 140, 127, 120, 92, 79]
new_year = np.linspace(2006, 2021, 150)
plt.plot(year, population, "ro")
for kind in ["nearest", "zero", "slinear", "quadratic", "cubic"]:
f = interpolate.interp1d(year, population, kind=kind)
new_population = f(new_year)
plt.plot(new_year, new_population, label=str(kind))
plt.legend(loc="lower right")
plt.show()
```
结果为:

注意,原代码使用的是 `pylab` 库,该库已经被弃用,建议使用 `matplotlib.pyplot` 库。另外,代码中的 `pl` 库应该改成 `plt` 库。
相关问题
# -*- coding: utf-8 -*- """ Created on Tue Apr 4 23:30:19 2023 @author: Json """ import matplotlib.pyplot as plt import numpy as np from matplotlib.animation import FuncAnimation fig = plt.figure() ax = fig.add_subplot(1, 1, 1) x = np.linspace(0, 2 * np.pi, 5000) y = np.exp(-x) * np.cos(2 * np.pi * x) line,= ax.plot(x, y, color="cornflowerblue", lw=3) ax.set_ylim(-1.1, 1.1) # # 清空当前帧 # def init(): # line.set_ydata([np.nan] * len(x)) # return line, #,init_func=init # 更新新一帧的数据 def update(frame): line.set_ydata(np.exp(-x) * np.cos(2 * np.pi * x + float(frame)/100)) return line, # 调用 FuncAnimation ani = FuncAnimation(fig ,update ,frames=200 ,interval=2 ,blit=True ) ani.save("animation.gif", fps=25, writer="imagemagick")
这段代码是一个 Python 的动画代码,使用了 Matplotlib 库进行绘图和动画展示。它定义了一个函数 init(),用于清空当前帧的数据。然后定义了一个 update() 函数,用于更新新一帧的数据。最后使用 Matplotlib 的 FuncAnimation() 函数创建了一个动画对象 ani,并保存为 GIF 格式的动画文件。
阅读全文
相关推荐



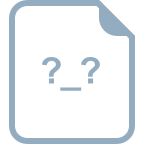




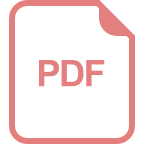



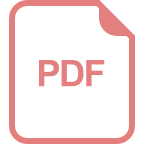




