写一份ffmpeg异步代码
时间: 2023-04-06 21:04:57 浏览: 104
以下是一份基于FFmpeg的异步代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/avutil.h>
#include <libavutil/opt.h>
#include <libavutil/imgutils.h>
#define THREAD_NUM 4
typedef struct {
AVFormatContext *fmt_ctx;
AVCodecContext *codec_ctx;
AVFrame *frame;
int video_stream_index;
int finished;
} DecodeContext;
typedef struct {
AVCodecContext *codec_ctx;
AVPacket *pkt;
int finished;
} EncodeContext;
void *decode_thread(void *arg) {
DecodeContext *ctx = (DecodeContext *)arg;
AVPacket pkt;
int ret;
while (av_read_frame(ctx->fmt_ctx, &pkt) >= 0) {
if (pkt.stream_index == ctx->video_stream_index) {
ret = avcodec_send_packet(ctx->codec_ctx, &pkt);
if (ret < 0) {
fprintf(stderr, "Error sending a packet for decoding\n");
break;
}
while (ret >= 0) {
ret = avcodec_receive_frame(ctx->codec_ctx, ctx->frame);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
break;
} else if (ret < 0) {
fprintf(stderr, "Error during decoding\n");
break;
}
// Do something with the decoded frame here
}
}
av_packet_unref(&pkt);
}
ctx->finished = 1;
return NULL;
}
void *encode_thread(void *arg) {
EncodeContext *ctx = (EncodeContext *)arg;
int ret;
while (!ctx->finished) {
ret = avcodec_send_frame(ctx->codec_ctx, NULL);
if (ret < 0) {
fprintf(stderr, "Error sending a frame for encoding\n");
break;
}
while (ret >= 0) {
ctx->pkt = av_packet_alloc();
ret = avcodec_receive_packet(ctx->codec_ctx, ctx->pkt);
if (ret == AVERROR(EAGAIN) || ret == AVERROR_EOF) {
av_packet_unref(ctx->pkt);
break;
} else if (ret < 0) {
fprintf(stderr, "Error during encoding\n");
break;
}
// Do something with the encoded packet here
av_packet_unref(ctx->pkt);
}
}
return NULL;
}
int main(int argc, char **argv) {
AVFormatContext *fmt_ctx = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVFrame *frame = NULL;
AVPacket pkt;
int video_stream_index = -1;
int ret, i;
if (argc < 2) {
fprintf(stderr, "Usage: %s <input file>\n", argv[0]);
return 1;
}
av_register_all();
avformat_network_init();
ret = avformat_open_input(&fmt_ctx, argv[1], NULL, NULL);
if (ret < 0) {
fprintf(stderr, "Could not open input file '%s'\n", argv[1]);
return 1;
}
ret = avformat_find_stream_info(fmt_ctx, NULL);
if (ret < 0) {
fprintf(stderr, "Could not find stream information\n");
return 1;
}
for (i = 0; i < fmt_ctx->nb_streams; i++) {
if (fmt_ctx->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
fprintf(stderr, "Could not find video stream\n");
return 1;
}
codec = avcodec_find_decoder(fmt_ctx->streams[video_stream_index]->codecpar->codec_id);
if (!codec) {
fprintf(stderr, "Codec not found\n");
return 1;
}
codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
fprintf(stderr, "Could not allocate codec context\n");
return 1;
}
ret = avcodec_parameters_to_context(codec_ctx, fmt_ctx->streams[video_stream_index]->codecpar);
if (ret < 0) {
fprintf(stderr, "Could not copy codec parameters to context\n");
return 1;
}
ret = avcodec_open2(codec_ctx, codec, NULL);
if (ret < 0) {
fprintf(stderr, "Could not open codec\n");
return 1;
}
frame = av_frame_alloc();
if (!frame) {
fprintf(stderr, "Could not allocate frame\n");
return 1;
}
pthread_t decode_threads[THREAD_NUM];
DecodeContext decode_contexts[THREAD_NUM];
for (i = 0; i < THREAD_NUM; i++) {
decode_contexts[i].fmt_ctx = fmt_ctx;
decode_contexts[i].codec_ctx = codec_ctx;
decode_contexts[i].frame = frame;
decode_contexts[i].video_stream_index = video_stream_index;
decode_contexts[i].finished = 0;
pthread_create(&decode_threads[i], NULL, decode_thread, &decode_contexts[i]);
}
pthread_t encode_threads[THREAD_NUM];
EncodeContext encode_contexts[THREAD_NUM];
for (i = 0; i < THREAD_NUM; i++) {
encode_contexts[i].codec_ctx = codec_ctx;
encode_contexts[i].pkt = NULL;
encode_contexts[i].finished = 0;
pthread_create(&encode_threads[i], NULL, encode_thread, &encode_contexts[i]);
}
for (i = 0; i < THREAD_NUM; i++) {
pthread_join(decode_threads[i], NULL);
}
for (i = 0; i < THREAD_NUM; i++) {
encode_contexts[i].finished = 1;
}
for (i = 0; i < THREAD_NUM; i++) {
pthread_join(encode_threads[i], NULL);
}
av_frame_free(&frame);
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
return 0;
}
```
这份代码使用了多线程来异步地进行解码和编码。在解码线程中,每个线程从输入文件中读取数据包并进行解码,解码后的帧可以在这里进行处理。在编码线程中,每个线程从解码线程中获取解码后的帧并进行编码,编码后的数据包可以在这里进行处理。这种异步的方式可以提高处理速度和效率。
相关推荐
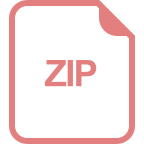
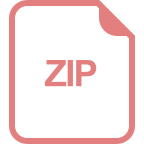
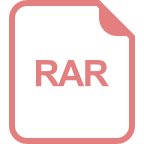















