I2 = Image.open('image/handwrite2v.jpg') I2_width, I2_height = I2.size for n in range(8): I1 = Image.open('image/hw01-gray.jpeg') I1_width, I1_height = I1.size for x in range(I1_width): for y in range(I1_height): I1_pixel = I1.getpixel((x, y)) if x < I2_width and y < I2_height: I2_pixel = I2.getpixel((x, y)) L = I2_pixel ^ (1 << n) if L != 0: # 说明是1 new_pixel = I1_pixel | L I1.putpixel((x, y), new_pixel) else: # 说明是0 new_pixel = I1_pixel | (1 << n) # 替换为1 new_pixel = new_pixel ^ (1 << n) # 替换为0 I1.save('image/hw-01-L' + str(n) + '.jpg') I1.close()能用numpy库等进行优化吗?
时间: 2023-12-04 19:06:16 浏览: 131
可以使用NumPy库对代码进行优化,从而提高运行效率。以下是使用NumPy库改写的代码示例:
```python
import numpy as np
from PIL import Image
# 读取原始图像和待修改图像
I1 = Image.open('image/hw01-gray.jpeg')
I2 = Image.open('image/handwrite2v.jpg')
# 将图像转换为NumPy数组
I1_arr = np.array(I1)
I2_arr = np.array(I2)
# 获取图像的宽度和高度
I1_height, I1_width = I1_arr.shape[:2]
I2_height, I2_width = I2_arr.shape[:2]
# 对每一位进行修改
for n in range(8):
# 计算需要修改的值
L = 1 << n
M = ~L
# 对每个像素进行处理
for x in range(I1_width):
for y in range(I1_height):
# 获取原始图像和待修改图像的像素值
I1_pixel = I1_arr[y, x]
if x < I2_width and y < I2_height:
I2_pixel = I2_arr[y, x]
else:
I2_pixel = 0
# 判断是否需要修改
if I2_pixel & L:
I1_pixel = (I1_pixel & M) | L
elif I2_pixel & M:
I1_pixel = (I1_pixel & M)
# 更新像素值
I1_arr[y, x] = I1_pixel
# 将修改后的图像保存到文件
I1_new = Image.fromarray(I1_arr)
I1_new.save('image/hw-01-L' + str(n) + '.jpg')
# 关闭文件
I1.close()
```
这个改写后的代码使用了NumPy数组,可以一次性处理图像数据,从而提高运行效率。同时,使用了位运算技巧来进行图像修改,也可以提高效率。
阅读全文
相关推荐
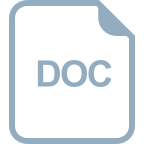
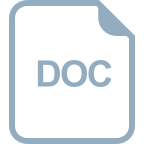
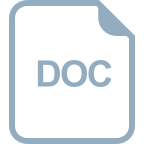
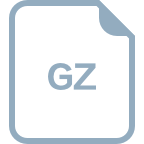
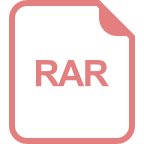
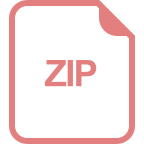
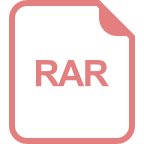
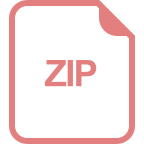
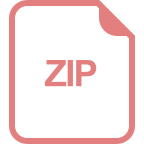
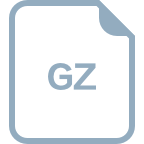
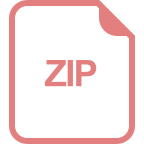
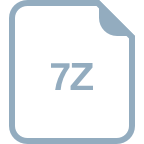
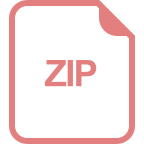
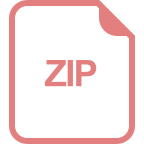
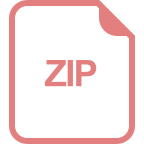