用C语言写该题目:给定一个可包含重复字母的序列,按任意顺序返回所有不重复的全排 列。
时间: 2024-04-30 09:23:28 浏览: 58
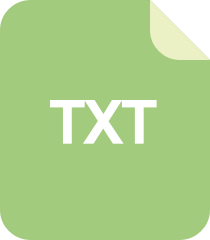
回溯法 - 输出自然数1到n所有不重复的排列,即n的全排列
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_N 100
void dfs(char *s, int len, int *used, char *path, int depth, char **res, int *resSize) {
if (depth == len) {
res[*resSize] = (char *)malloc(sizeof(char) * (len + 1));
strcpy(res[*resSize], path);
(*resSize)++;
return;
}
for (int i = 0; i < len; i++) {
if (used[i] || (i > 0 && s[i] == s[i - 1] && !used[i - 1])) {
continue;
}
path[depth] = s[i];
used[i] = 1;
dfs(s, len, used, path, depth + 1, res, resSize);
used[i] = 0;
}
}
char **permuteUnique(char *s, int *returnSize) {
int len = strlen(s);
int used[MAX_N] = {0};
char path[MAX_N] = {0};
char **res = (char **)malloc(sizeof(char *) * MAX_N * MAX_N);
*returnSize = 0;
// 排序是为了剪枝
qsort(s, len, sizeof(char), strcmp);
dfs(s, len, used, path, 0, res, returnSize);
return res;
}
int main() {
char *s = "abc";
int returnSize;
char **res = permuteUnique(s, &returnSize);
for (int i = 0; i < returnSize; i++) {
printf("%s\n", res[i]);
}
return 0;
}
```
阅读全文
相关推荐
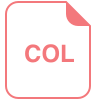
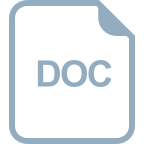
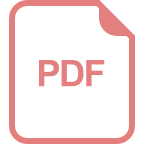
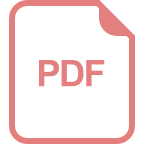
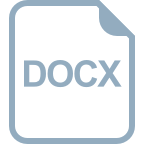
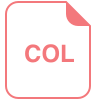
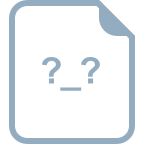
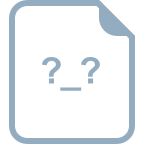
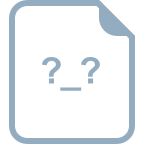
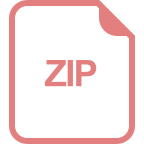
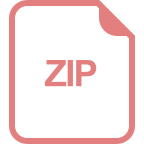
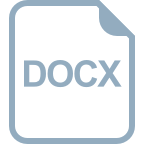