mybatis中模糊查询方式
时间: 2023-05-19 20:06:55 浏览: 138
有两种模糊查询方式可以在 MyBatis 中使用:使用通配符的模糊查询和使用正则表达式的模糊查询。使用通配符的模糊查询可以使用 % 或 _ 通配符,其中 % 表示任意字符出现任意次数,_ 表示任意单个字符。使用正则表达式的模糊查询需要使用 MyBatis 提供的正则表达式函数进行匹配。
相关问题
mybatis 模糊查询
MyBatis 中的模糊查询可以使用 SQL 中的 `LIKE` 关键字实现。
例如,假设我们有一个表 `user`,其中有一个名为 `name` 的字段,我们想要模糊查询名字中包含关键字 `Tom` 的用户,可以使用以下 SQL 语句:
```sql
SELECT * FROM user WHERE name LIKE '%Tom%'
```
在 MyBatis 中,我们可以使用 XML 映射文件或注解方式来实现该查询,如下所示:
XML 映射文件:
```xml
<select id="selectUsersByName" resultType="User">
SELECT * FROM user WHERE name LIKE CONCAT('%', #{name}, '%')
</select>
```
注解方式:
```java
@Select("SELECT * FROM user WHERE name LIKE CONCAT('%', #{name}, '%')")
List<User> selectUsersByName(String name);
```
其中,`#{name}` 表示使用 MyBatis 参数占位符的方式来代替 SQL 中的参数,防止 SQL 注入攻击。`CONCAT()` 函数用于将 `%` 和参数拼接起来,构成模糊查询的关键字。
myBatis模糊查询
使用Spring Boot和MyBatis进行模糊查询非常简单。您可以使用MyBatis的动态SQL功能来构建模糊查询语句。
以下是一个示例:
```java
@Mapper
public interface UserMapper {
@Select("SELECT * FROM users WHERE name LIKE CONCAT('%',#{name},'%')")
List<User> findUsersByName(String name);
}
```
在上面的示例中,我们使用了MyBatis的动态SQL功能来构建一个模糊查询语句。我们使用了`CONCAT`函数来将`%`符号与查询参数`name`连接起来,从而构建了一个模糊查询语句。
您可以在Spring Boot应用程序中使用这个Mapper接口来执行模糊查询。例如:
```java
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public List<User> findUsersByName(String name) {
return userMapper.findUsersByName(name);
}
}
```
在上面的示例中,我们注入了`UserMapper`接口,并在`UserService`中使用它来执行模糊查询。
阅读全文
相关推荐
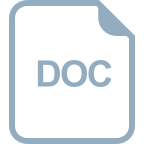
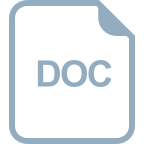
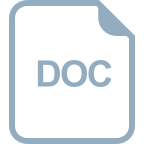
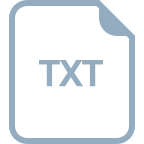



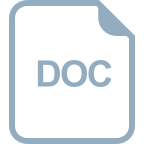
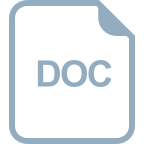
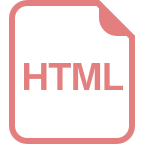