编写一群试图并行解决任务的爬山者
时间: 2023-06-10 18:06:38 浏览: 142
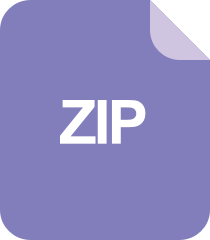
定时任务quartz实现分组串行并行动态配置
好的,我可以为你提供一个简单的并行爬山算法实现,用于解决多个起始点的最小化问题。
算法步骤如下:
1. 初始化并行爬山者的数量和迭代次数。
2. 随机生成若干个起始点,每个起始点代表一个爬山者。
3. 对于每个爬山者,以它的起始点作为初始状态,执行一次传统的爬山算法,求出该爬山者的最优解。
4. 将每个爬山者的最优解汇总,选择其中最优的一个作为当前的全局最优解。
5. 对于每个爬山者,以当前全局最优解作为初始状态,继续执行一次传统的爬山算法,求出该爬山者的最优解。
6. 将每个爬山者的最优解汇总,选择其中最优的一个作为当前的全局最优解。
7. 重复步骤5和6,直到迭代次数达到设定值。
下面是一个可能的实现,使用Python语言和multiprocessing库:
```python
import random
import multiprocessing
# 定义目标函数,这里以一个简单的二维函数为例
def target_func(x, y):
return x ** 2 + y ** 2
# 定义爬山算法,这里使用随机爬山算法
def hill_climbing(start_point):
current_point = start_point
current_value = target_func(*current_point)
while True:
next_points = [(current_point[0] + random.uniform(-1, 1), current_point[1] + random.uniform(-1, 1))
for _ in range(10)]
next_values = [target_func(*p) for p in next_points]
next_point, next_value = min(zip(next_points, next_values), key=lambda x: x[1])
if next_value < current_value:
current_point = next_point
current_value = next_value
else:
break
return current_point, current_value
# 定义并行爬山函数
def parallel_hill_climbing(num_workers, num_iterations, start_points):
global_best_point, global_best_value = None, float('inf')
pool = multiprocessing.Pool(num_workers)
for i in range(num_iterations):
results = pool.map(hill_climbing, start_points)
best_point, best_value = min(results, key=lambda x: x[1])
if best_value < global_best_value:
global_best_point, global_best_value = best_point, best_value
start_points = [global_best_point] * len(start_points)
return global_best_point, global_best_value
# 测试代码
if __name__ == '__main__':
num_workers = 4
num_iterations = 10
start_points = [(random.uniform(-10, 10), random.uniform(-10, 10)) for _ in range(num_workers)]
best_point, best_value = parallel_hill_climbing(num_workers, num_iterations, start_points)
print(f'Best point: {best_point}, best value: {best_value}')
```
在这个实现中,我们使用了Python的multiprocessing库来实现并行化。在并行爬山函数`parallel_hill_climbing`中,我们首先创建一个进程池,并将起始点列表`start_points`分配给进程池中的各个进程。每个进程执行一次`hill_climbing`函数,得到该进程的最优解。最后,我们汇总每个进程的最优解,并选择其中最优的一个作为当前的全局最优解。我们将全局最优解作为起始点,继续执行`hill_climbing`函数,得到新的最优解。重复这个过程,直到达到设定的迭代次数。最终,算法返回的是全局最优解和对应的函数值。
需要注意的是,由于并行化的实现涉及到进程间的数据共享和通信,所以在实现时需要格外注意数据的同步和保护,以免出现意外的错误。
阅读全文
相关推荐
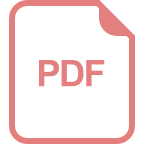
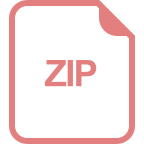
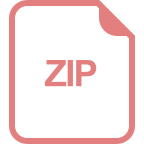
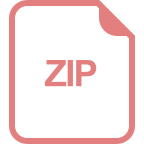
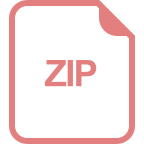
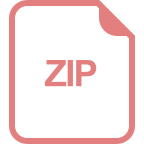
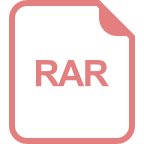
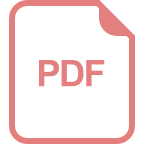
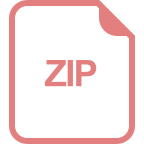
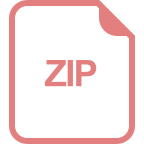
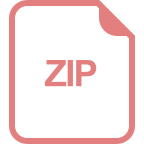
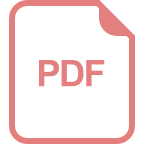
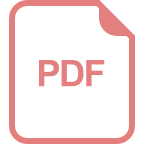
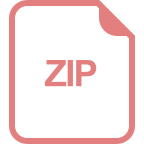
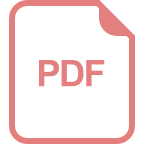
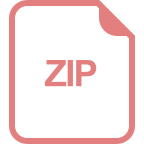