springboot文件上传mysql
时间: 2023-10-14 09:30:55 浏览: 87
实现文件上传到 MySQL 数据库可以采用以下步骤:
1. 配置 pom.xml 文件,引入所需的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<!-- 文件上传 -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.3.1</version>
</dependency>
<dependency>
<groupId>commons-io</groupId>
<artifactId>commons-io</artifactId>
<version>2.4</version>
</dependency>
```
2. 配置数据库连接信息,在 application.properties 文件中添加如下配置:
```properties
spring.datasource.url=jdbc:mysql://localhost:3306/test?characterEncoding=utf8&useSSL=false
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# Hibernate ddl auto (create, create-drop, validate, update)
spring.jpa.hibernate.ddl-auto=update
spring.jpa.show-sql=true
# 文件上传大小限制
spring.servlet.multipart.max-request-size=10MB
spring.servlet.multipart.max-file-size=10MB
```
3. 创建文件上传的 Controller:
```java
@Controller
public class FileUploadController {
@Autowired
private FileRepository fileRepository;
@GetMapping("/")
public String index(Model model) {
List<MyFile> files = fileRepository.findAll();
model.addAttribute("files", files);
return "index";
}
@PostMapping("/upload")
public String upload(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) {
if (file.isEmpty()) {
redirectAttributes.addFlashAttribute("message", "Please select a file to upload");
return "redirect:/";
}
try {
// 获取文件名
String fileName = StringUtils.cleanPath(file.getOriginalFilename());
MyFile myFile = new MyFile(fileName, file.getContentType(), file.getBytes());
fileRepository.save(myFile);
redirectAttributes.addFlashAttribute("message", "You successfully uploaded " + fileName + '!');
} catch (IOException e) {
e.printStackTrace();
}
return "redirect:/";
}
}
```
4. 创建实体类 MyFile:
```java
@Entity
@Table(name = "my_file")
public class MyFile {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String type;
@Lob
private byte[] data;
public MyFile() {
}
public MyFile(String name, String type, byte[] data) {
this.name = name;
this.type = type;
this.data = data;
}
// getters and setters
}
```
5. 创建 Repository 接口:
```java
public interface FileRepository extends JpaRepository<MyFile, Long> {
}
```
6. 创建页面模板 index.html:
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>File Upload</title>
</head>
<body>
<h2>File Upload</h2>
<form th:action="@{/upload}" method="post" enctype="multipart/form-data">
<input type="file" name="file"/><br/><br/>
<input type="submit" value="Upload"/>
</form>
<p th:text="${message}"></p>
<h2>Files</h2>
<table>
<thead>
<tr>
<th>Name</th>
<th>Type</th>
<th>Download</th>
</tr>
</thead>
<tbody>
<tr th:each="file : ${files}">
<td th:text="${file.name}"></td>
<td th:text="${file.type}"></td>
<td><a th:href="@{/download/{id}(id=${file.id})}">Download</a></td>
</tr>
</tbody>
</table>
</body>
</html>
```
7. 创建文件下载的 Controller:
```java
@Controller
public class FileDownloadController {
@Autowired
private FileRepository fileRepository;
@GetMapping("/download/{id}")
public ResponseEntity<byte[]> download(@PathVariable Long id) {
Optional<MyFile> optionalMyFile = fileRepository.findById(id);
if (optionalMyFile.isPresent()) {
MyFile myFile = optionalMyFile.get();
HttpHeaders headers = new HttpHeaders();
headers.setContentDispositionFormData("attachment", myFile.getName());
headers.setContentType(MediaType.parseMediaType(myFile.getType()));
return new ResponseEntity<>(myFile.getData(), headers, HttpStatus.OK);
}
return new ResponseEntity<>(HttpStatus.NOT_FOUND);
}
}
```
至此,文件上传到 MySQL 数据库的实现就完成了。
阅读全文
相关推荐
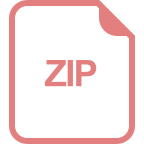
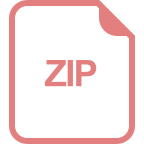
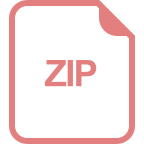














