使用selenium登录立可得到后台(http://likede2-admin.itheima.net/#/login?redirect=%2Fhome)并且使用百度ocr识别立可得登陆页面中的字符验证码
时间: 2024-01-01 16:06:10 浏览: 23
好的,使用 Python 和 Selenium 可以模拟登录立可得后台并使用百度 OCR 识别验证码。首先需要安装相应的库,比如 `selenium`、`requests`、`Pillow` 和 `baidu-aip`。
以下是代码示例:
```python
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
import time
from PIL import Image
import requests
import base64
from aip import AipOcr
# 设置百度 OCR API 的 APP ID、API Key 和 Secret Key
APP_ID = 'your_app_id'
API_KEY = 'your_api_key'
SECRET_KEY = 'your_secret_key'
# 创建 AipOcr 对象并设置相关参数
client = AipOcr(APP_ID, API_KEY, SECRET_KEY)
client.setConnectionTimeoutInMillis(5000)
client.setSocketTimeoutInMillis(10000)
# 创建浏览器对象,打开立可得后台登录页面
browser = webdriver.Chrome()
browser.get('http://likede2-admin.itheima.net/#/login?redirect=%2Fhome')
# 等待页面加载完成
time.sleep(3)
# 定位用户名和密码输入框,并输入用户名和密码
username_input = browser.find_element_by_css_selector('.el-input__inner[type="text"]')
username_input.send_keys('your_username')
password_input = browser.find_element_by_css_selector('.el-input__inner[type="password"]')
password_input.send_keys('your_password')
# 定位验证码图片并下载图片
code_img = browser.find_element_by_css_selector('.code-img img')
code_img_src = code_img.get_attribute('src')
response = requests.get(code_img_src)
with open('code.png', 'wb') as f:
f.write(response.content)
# 使用百度 OCR 识别验证码
with open('code.png', 'rb') as f:
image = f.read()
result = client.basicGeneral(image)
if 'words_result' in result:
code = result['words_result'][0]['words']
print('识别结果为:', code)
else:
print('识别失败')
# 输入验证码并模拟点击登录按钮
code_input = browser.find_element_by_css_selector('.el-input__inner[type="tel"]')
code_input.send_keys(code)
login_btn = browser.find_element_by_css_selector('.login-btn')
login_btn.click()
# 等待页面加载完成
time.sleep(3)
# 关闭浏览器
browser.quit()
```
需要注意的是,百度 OCR API 每天有免费的调用次数限制,超过限制后需要付费。此外,验证码可能会有多种类型,识别效果可能会有所不同。
相关推荐
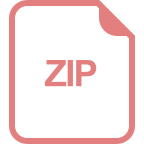
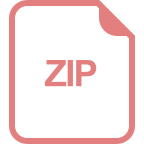
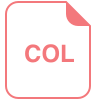
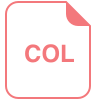
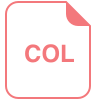
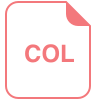
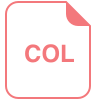









